Missing default argument - compiler error
Solution 1
You can't have non-default parameters after your default parameters begin. Put another way, how would you specify a value for b
leaving word
to the default of "hello" ?
Solution 2
The arguments with a default value have to come in the end of the argument list.
So just change your function declaration to
void func(int b, string word = "hello")
Solution 3
Parameters with default values have to come at the end of the list because, when calling the function, you can leave arguments off the end, but can't miss them out in the middle.
Since your arguments have different types, you can get the same effect using an overload:
void func ( string word, int b ) {
// some jobs
}
void func ( int b ) { func("hello", b); }
Solution 4
The error message is proper. If the default argument is assigned to a given parameter then all subsequent parameters should have a default argument. You can fix it in 2 ways;
(1) change the order of the argument:
void func (int b, string word = "hello");
(2) Assign a default value to b
:
void func (string word = "hello", int b = 0);
Solution 5
You cannot fix it without changing anything!
To fix it, you can use overloading:
void func ( string word, int b ) {
// some jobs
}
void func ( string word ) {
func( word, 999 );
}
void func ( int b ) {
func( "hello", b );
}
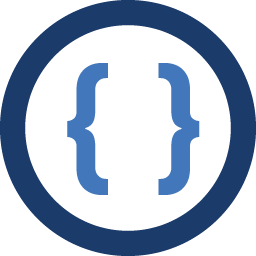
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
void func ( string word = "hello", int b ) { // some jobs } in another function //calling func ( "", 10 ) ;
When I have compiled it, compiler emits error ;
default argument missing for parameter
How can I fix it without changing anything, of course, such as not making "int b = 0" ? Moreover, I want use that function like func ( 10 ) or func ( "hi" ) ? Is my compiler not do its job, properly ?
-
Matthieu M. about 13 years+1 for suggesting the overload, it achieves exactly the calling syntax that defaulted middle arguments would.