missing permissions required by BluetoothAdapter.isEnabled.BLUETOOTH
Solution 1
- You only need
<uses-permission>
tag since you are using a permission. You don't need<permissions>
tag since this is not your custom permission. - Maybe you have placed
<uses-permission>
tag in the wrong element in manifest, but I cannot say anything else with the information you provided - Since your bluetoothAdapter can be null, either add to second IF null pointer check, or add a RETURN in first IF
- It is also nice to have
<uses-feature>
tag
Solution 2
Let's say you have a button and then you click on it, Bluetooth needs to turn on. Here's a quick example how you would do it for Android 6.0 and above.
First, declare this variable in your Activity
/Fragment
:
public static final int PERMISSION_ASK = 1001;
Now, let's say this buttons is going to enable the bluetooth.
setOnClickListener
:
myButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
if(isBluetoothPermissionGranted()) {
// app already has required permissions
// do some task here
} else {
// app does not have permission yet.
// ask for permissions
askForBluetoothPermissions();
}
} else {
// Android version below 6.0, no need to check or ask for permission
// do some task here
}
}
});
Checking if application already has the required permission:
@RequiresApi(api = Build.VERSION_CODES.M)
private boolean isBluetoothPermissionGranted() {
boolean granted = false;
int bluetoothGranted = checkSelfPermission(Manifest.permission.BLUETOOTH);
int bluetoothAdminGranted = checkSelfPermission(Manifest.permission.BLUETOOTH_ADMIN);
if(bluetoothGranted == PackageManager.PERMISSION_GRANTED &&
bluetoothAdminGranted == PackageManager.PERMISSION_GRANTED) {
granted = true;
}
return granted;
}
If required permission is not granted, here's how you would ask for it:
@RequiresApi(api = Build.VERSION_CODES.M)
private void askForBluetoothPermissions() {
String[] permissions = new String[] {
Manifest.permission.BLUETOOTH,
Manifest.permission.BLUETOOTH_ADMIN
};
requestPermissions(permissions, PERMISSION_ASK);
}
And finally, here's how you would confirm if the user granted you the permission(s) or not:
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
switch (requestCode) {
case PERMISSION_ASK:
if(grantResults[0] == PackageManager.PERMISSION_GRANTED && grantResults[1] == PackageManager.PERMISSION_GRANTED) {
// all requested permissions were granted
// perform your task here
} else {
// permissions not granted
// DO NOT PERFORM THE TASK, it will fail/crash
}
break;
default:
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
break;
}
}
Hope this helps
Solution 3
For Android 6.0 and above you must add the following to manifest file
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<permission android:name="android.permission.BLUETOOTH" android:label="BLUETOOTH" />
<permission android:name="android.permission.BLUETOOTH_ADMIN" />
<permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
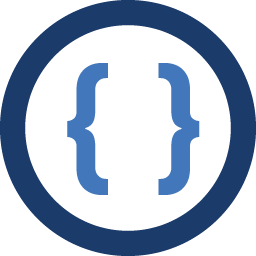
Admin
Updated on June 24, 2022Comments
-
Admin about 2 years
I add bluetooth to my app but am running into the following problem. When I do the code:
BluetoothAdapter bluetoothAdapter=BluetoothAdapter.getDefaultAdapter(); if (bluetoothAdapter == null) { Toast.makeText(getApplicationContext(),"Device doesnt Support Bluetooth",Toast.LENGTH_SHORT).show(); } if(!bluetoothAdapter.isEnabled()) { Intent enableAdapter = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); startActivityForResult(enableAdapter, 0); }
The error is on the following line:
if(!bluetoothAdapter.isEnabled())
Error:
Missing permissions required by BluetoothAdapter.isEnabled :android.permissions.BLUETOOTH
I added the following permissions to the android manifest file:
<uses-permission android:name="android.permission.BLUETOOTH" /> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" /> <permission android:name="android.permission.BLUETOOTH" android:label="BLUETOOTH" /> <permission android:name="android.permission.BLUETOOTH_ADMIN" />
but am still getting the same issue. Do you know what I did wrong?