Module with Main dispatcher is missing
Solution 1
Using just the kotlinx-coroutines-android version solves the problem.
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:0.30.1'
Solution 2
You may be missing some Proguard rules.
I had the same problem in release build and solved it by adding the following rules:
-keepnames class kotlinx.coroutines.internal.MainDispatcherFactory {}
-keepnames class kotlinx.coroutines.CoroutineExceptionHandler {}
-keepclassmembernames class kotlinx.** {
volatile <fields>;
}
Solution 3
Use both Core and Android dependency
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-core:1.3.6'
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.3.6'
Solution 4
If you're facing this problem with coroutines version 1.3.8, add this rule in your proguard-rules.pro
:
-keep class kotlinx.coroutines.android.AndroidDispatcherFactory {*;}
Solution 5
./gradlew assembleDebug --rerun-tasks
fixes it if the above answers don't work for you (because you already had the required dependencies and you are using R8 which does not need the proguard rules).
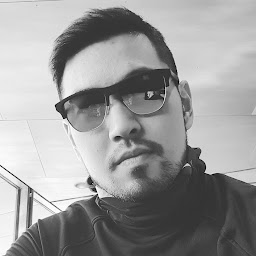
Khongor Bayarsaikhan
Android developer, interested in new technologies.
Updated on July 09, 2022Comments
-
Khongor Bayarsaikhan almost 2 years
I'm trying to make a background call to my local database and update the UI with the results using coroutines. Here is my relevant code:
import kotlinx.coroutines.experimental.* import kotlinx.coroutines.experimental.Dispatchers.IO import kotlinx.coroutines.experimental.Dispatchers.Main import kotlin.coroutines.experimental.CoroutineContext import kotlin.coroutines.experimental.suspendCoroutine class WarehousesViewModel(private val simRepository: SimRepository) : BaseReactViewModel<WarehousesViewData>(), CoroutineScope { private val job = Job() override val coroutineContext: CoroutineContext get() = job + Main override val initialViewData = WarehousesViewData(emptyList()) override fun onActiveView() { launch { val warehouses = async(IO) { loadWarehouses() }.await() updateViewData(viewData.value.copy(items = warehouses)) } } private suspend fun loadWarehouses(): List<Warehouse> = suspendCoroutine {continuation -> simRepository.getWarehouses(object : SimDataSource.LoadWarehousesCallback { override fun onWarehousesLoaded(warehouses: List<Warehouse>) { Timber.d("Loaded warehouses") continuation.resume(warehouses) } override fun onDataNotAvailable() { Timber.d("No available data") continuation.resume(emptyList()) } }) } }
My problem is that I get a runtime exception:
java.lang.IllegalStateException: Module with Main dispatcher is missing. Add dependency with required Main dispatcher, e.g. 'kotlinx-coroutines-android'
I already added these to my gradle:
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-core:0.30.1' implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:0.26.0'
I'm a bit new to this, can someone help me?
-
Marko Topolnik over 5 years
kotlinx-coroutines-core
is a transitive dependency ofkotlinx-coroutines-android
and the proper solution is to remove it. -
Lucas P. about 5 yearsThat was it for my case. I've added all of the keeps form the GitHub page you've provided. Thank you very much!
-
Herbal7ea almost 5 yearsGood catch Marko! Just to clarify, Marko means remove
kotlinx-coroutines-core
, it's included inkotlinx-coroutines-android
-
Alexei almost 5 yearsTo be use in Kotlin 1.3 must use this implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.2.1'
-
Pierry over 4 yearsI've just change for my flavor and all right! thanks
-
cren90 over 4 yearsThe GitHub page linked is no longer available, 404's for me
-
Ganesh Kanna over 4 yearsIt doesn't matter cren90 . I want to share as example. You can simply follow what I mentioned
-
Khongor Bayarsaikhan almost 4 yearsas pointed below the accepted answer, using only the android is enough
-
Alessandro Mautone over 3 yearsI run into this as well and indeed seems like the
AndroidDispatcherFactory
was not deobfuscated properly. With this in the proguard rules it works -
DJ. Aduvanchik over 3 yearsimplementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.3.3' - is the most current if anyone wants to save a few seconds.
-
Damn Vegetables over 2 years@MarkoTopolnik I am confused. It is NOT an Android project, but a plain Kotlin console project (the one that prints "hello world" in the command prompt), and I have added
org.jetbrains.kotlinx:kotlinx-coroutines-core-jvm:1.6.0
as another answer had suggested, and I encountered this runtime error when trying to useDispatchers.Main
. So, I should usekotlinx-coroutines-android
even if it is not an Android project?kotlinx-coroutines-core-jvm
has been deprecated and should no longer be used? -
Damn Vegetables over 2 years@MarkoTopolnik I have tried switching to Android version, but it threw a new runtime exception: NoClassDefFoundError: android/os/Looper
-
Marko Topolnik over 2 years@DamnVegetables Indeed, don't try to use either
kotlinx-coroutines-android
orDispatchers.Main
outside of an Android application.