Most efficient way of adding/removing a character to beginning of string?
Solution 1
Both are equally efficient I think since both require a new string
to be initialized, since string
is immutable.
When doing this on the same string multiple times, a StringBuilder
might come in handy when adding. That will increase performance over adding.
You could also opt to move this operation to the database side if possible. That might increase performance too.
Solution 2
If you have several records and to each of the several records field you need to append a character at the beginning, you can use String.Insert
with an index of 0 http://msdn.microsoft.com/it-it/library/system.string.insert(v=vs.110).aspx
string yourString = yourString.Insert( 0, "C" );
This will pretty much do the same of what you wrote in your original post, but since it seems you prefer to use a Method and not an operator...
If you have to append a character several times, to a single string, then you're better using a StringBuilder
http://msdn.microsoft.com/it-it/library/system.text.stringbuilder(v=vs.110).aspx
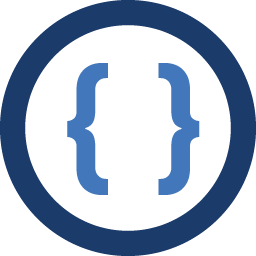
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I was doing a small 'scalable' C# MVC project, with quite a bit of read/write to a database.
From this, I would need to add/remove the first letter of the input string.
'Removing' the first character is quite easy (using a Substring method) - using something like:
String test = "HHello world"; test = test.Substring(1,test.Length-1);
'Adding' a character efficiently seems to be messy/awkward:
String test = "ello World"; test = "H" + test;
Seeing as this will be done for a lot of records, would this be be the most efficient way of doing these operations?
I am also testing if a string starts with the letter 'T' by using, and adding 'T' if it doesn't by:
String test = "Hello World"; if(test[0]!='T') { test = "T" + test; }
and would like to know if this would be suitable for this