multi line textbox to array C#
Solution 1
You could use a List instead of a string array Then the AddRange method could simplify your method eliminatig the foreach loop
List<string> ParkingTimes = new List<string>()
ParkingTimes.Add(tbxtimeLimitS1.Text);
ParkingTimes.AddRange(tbxparkingTimesS1.Lines);
ParkingTimes.AddRange(tbxtimeLimitS2.Lines);
ParkingTimes.AddRange(tbxparkingTimesS2.Lines);
ParkingTimes.AddRange(tbxtimeLimitS2.Lines);
ParkingTimes.AddRange(tbxparkingTimesS3.Lines);
If your code still requires a string array it is possible to get back the array with
string[] myLines = ParkingTimes.ToArray();
An example of this List<string>
functionality could be found on MSDN here
Solution 2
You can simply do
string[] allLines = textbox.Text.Split('\n');
This will split each line and store the results in the appropriate index in the array. You can then iterate over them like so:
foreach (string text in allLines)
{
//do whatever with text
}
Solution 3
You can do something like this:
var totalLines = new List<String>();
totalLines.AddRange( tbxparkingTimesS1.Lines );
totalLines.AddRange( tbxparkingTimesS2.Lines );
totalLines.AddRange( tbxparkingTimesS3.Lines );
if you need it in an array instead of a list, then call:
var array = totalLines.ToArray();
Hope it helps.
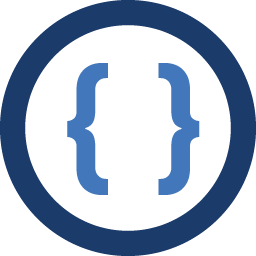
Admin
Updated on October 26, 2020Comments
-
Admin over 3 years
I am trying to transfer values from each line of a multi line text box into either a string array or a multidimensional array. I also have 3 multi-line text boxes which need to put into the same array. Below is one of the methods I have been trying:
ParkingTimes[0] = tbxtimeLimitS1.Text; for (int i = 1; i <= 10; i++) ParkingTimes[i] = tbxparkingTimesS1.Lines; ParkingTimes[11] = tbxtimeLimitS2.Lines; for (int x = 0; x <= 10; x++) for (int i = 12; i <= 21; i++) ParkingTimes[i] = tbxparkingTimesS2.Lines; ParkingTimes[11] = tbxtimeLimitS2.Lines[0]; for (int x = 0; x <= 10; x++) for (int i = 23; i <= 32; i++) ParkingTimes[i] = tbxparkingTimesS3.Lines;
What am I doing wrong? Is there a better way to accomplish this?
-
Admin almost 12 yearshow do i add add the text in allLines to a string array?
-
Admin almost 12 yearshow do i add the line to the array in the foreach loop?
-
Nick Udell almost 12 yearsallLines will be your string array. The output of textbox.Text.Split() should be an array of strings, which would then be assigned to allLines
-
Bryan Crosby almost 12 years@JackTcRebelTreble: Yes I did mean .Text.Split(), sorry. @Nick Udell is correct: The
allLines
variable is the array with the strings. -
Admin almost 12 yearsThank You! - just testing it out now