Multiple Rest calls in Java using RestAssured
10,448
Completable Future can be used to make calls async.
List<CompletableFuture<Pair<String,String>>> futures =
Arrays.stream(endpoints)
.map(endpoint -> restCallAsync(endpoint))
.collect(Collectors.toList());
List<Pair<String,String>> result =
futures.stream()
.map(CompletableFuture::join)
.collect(Collectors.toList());
CompletableFuture<Pair<String,String>> restCallAsync(String endpoint) {
CompletableFuture<Pair<String,String>> future = CompleteableFuture.supplyAsync(new Supplier<Pair<String,String>>() {
@Override
public Pair<String,String> get() {
Response response = given()
.relaxedHTTPSValidation()
.get(endpoint)
.then()
.extract().response();
LOG.info("Endpoint : " + endpoint + " Status : " + response.getStatusCode());
...
}
});
}
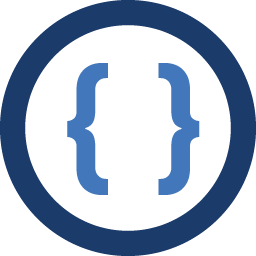
Author by
Admin
Updated on November 29, 2022Comments
-
Admin over 1 year
When you make a rest request using RestAssured, it appears to wait for a response. I need to make a POST request in RestAssured and then while it waits for a response, I need to make a GET request. I'm using Java and RestAssured. I've also tried creating a second thread and it still has not worked. This is for testing purposes.
Here's where it waits:
given().auth().preemptive().basic(userCreds[0], userCreds[1]).contentType("application/json;").and().post(baseURL + resourcePath + this.act.getId() + "/run");
I would like this to run while the previous request is running as well (asynchronous request?):
given().auth().preemptive().basic(userCreds[0], userCreds[1]).when().get(baseURL + resourcePath + this.connect.getId() + "/outgoing/" + fileId);
I've also read that RestAssured supports asynchronous requests, but I've been unsuccessful to get it to work. My project is mavenized. I'm just a lowly QA guy so any help would be greatly appreciated.
-
Vitali Plagov over 5 yearsDo I have to use the same number of threads in method
newFixedThreadPool()
and infor
-loop? -
avp over 5 years@VitaliiPlagov No, you need not. They can be different based on your use case of whether you want to run it in caller's thread or a new thread.
-
Amrit over 3 yearsHi @TT, This is code snapshot for my private repository.
-
TT. over 3 yearsHi Amrit. Better to post the code as text instead a picture. Pictures cannot be searched and indexed from search engines.
-
Amrit over 3 yearsSure @TT will keep this in mind going forward, but this isn't copied from anywhere.