Multiply string in Java
Solution 1
Try this:
String buildString(char c, int n) {
char[] arr = new char[n];
Arrays.fill(arr, c);
return new String(arr);
}
Arrays.fill()
is a standard API method that assigns the given value to every element of the given array. I guess technically it'll be looping 'inside', in order to achieve this, but from the perspective of your program you've avoided looping.
Solution 2
While Java does not have a built in method of doing this, you can very easily accomplish this with a few lines of code using a StringBuilder
.
public static void main(String[] args) throws Exception {
System.out.println(stringMultiply("a", 2));
}
public static String stringMultiply(String s, int n){
StringBuilder sb = new StringBuilder();
for(int i = 0; i < n; i++){
sb.append(s);
}
return sb.toString();
}
The function stringMultiply
above does essentially the same thing. It will loop n
times (the number to multiply) and append the String
s
to the StringBuilder
each loop. My assumption is that python
uses the same logic for this, it is just a built in functionality, so your timing should not be too much different writing your own function
.
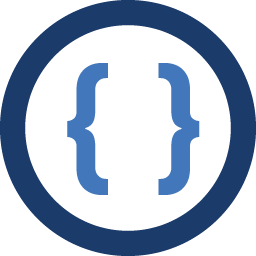
Admin
Updated on June 28, 2020Comments
-
Admin almost 4 years
In Python I can do this: print("a" * 2);
Output = aa
Is there a way in Java to do the same without loops?