Multiplying strings in bash script
12,356
Solution 1
You can use bash
command substitution
to be more portable across systems than to use a variant specific command.
$ myString=$(printf "%10s");echo ${myString// /m} # echoes 'm' 10 times
mmmmmmmmmm
$ myString=$(printf "%10s");echo ${myString// /rep} # echoes 'rep' 10 times
reprepreprepreprepreprepreprep
Wrapping it up in a more usable shell-function
repeatChar() {
local input="$1"
local count="$2"
printf -v myString "%s" "%${count}s"
printf '%s\n' "${myString// /$input}"
}
$ repeatChar str 10
strstrstrstrstrstrstrstrstrstr
Solution 2
You could simply use loop
$ for i in {1..4}; do echo -n 'm'; done
mmmm
Solution 3
In bash you can use simple string indexing in a similar manner
#!/bin/bash
oos="oooooooooooooo"
n=2
printf "%c%s\n" 'f' ${oos:0:n}
output
foo
Another approach simply concatenates characters into a string
#!/bin/bash
n=2
chr=o
str=
for ((i = 0; i < n; i++)); do
str="$str$chr"
done
printf "f%s\n" "$str"
Output
foo
There are several more that can be used as well.
Solution 4
That will do:
printf 'f'; printf 'o%.0s' {1..2}; echo
Look here for explanations on the "multiplying" part.
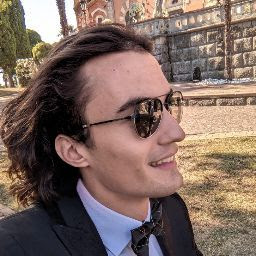
Author by
Jakob Kenda
Updated on June 09, 2022Comments
-
Jakob Kenda almost 2 years
I know that if I do
print ("f" + 2 * "o")
in python the output will befoo
.But how do I do the same thing in a bash script?
-
gniourf_gniourf over 6 yearsUse
printf -v myString '%*s' "$count"
instead ofmyString=$(...)
. And uselocal
to mark your variables local (or drop the local variables and use the parameters directly). -
Inian over 6 years@gniourf_gniourf: Yup, it is a pretty old answer. Will make the change anyway!
-
James T. over 2 yearsYou can simplify to
$ ${$(printf %10s)// /rep}
-
kvantour about 2 yearsI like this answer most, but I would rewrite it as:
printf -- "f%s\n" $(printf -- "o%.0s" {1..2})
. The downside is that it needs a subshell, but it does the job quite well. -
Inian about 2 years@pjh: Fixed it, turned out to be a wrong edit
-
pjh about 2 years
repeatChar
works now. Its handling of counts with leading zeros may be surprising to some though. (repeatChar str 09
fails with aninvalid octal number
error.)