MVC 4 View reload with change in data
I suppose that you wrote some javascript code so that when the user clicks on a <li>
element of the <ul>
you are triggering an AJAX call to the [HttpPost]
action sending the selected value to the server. The problem with your code might be that you probably forgot to update the DOM with the new contents in your success
callback. So for this to work you could start by placing the <ul>
contents in a partial view:
List.cshtml
:
@model IEnumerable<string>
@foreach (var i in Model)
{
<li>@Html.DisplayFor(ir=>i)</li>
}
and then include this partial in the main view:
@model IEnumerable<string>
@{
ViewBag.Title = "Index";
}
@using (Html.BeginForm())
{
@Html.ValidationSummary(true)
<h2>Index</h2>
<ul id="bb">
@Html.Partial("_List", Model)
</ul>
}
OK, now in your POST action you could return the partial:
[HttpPost]
public ActionResult Index(string value)
{
List<string> ll = new List<string>();
ll.Add("kkk");
ll.Add("qq");
ll.Add("aa");
ll.Add("zz");
return PartialView("_List", ll);
}
and the final bit is the javascript code:
$(function() {
$('#bb').on('click', 'li', function() {
var value = $(this).html();
$.ajax({
url: $(this).closest('form').attr('action'),
type: 'POST',
data: { value: value },
success: function(partialView) {
$('#bb').html(partialView);
}
});
});
});
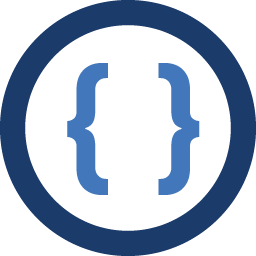
Admin
Updated on September 02, 2022Comments
-
Admin over 1 year
I have a simple controller and view:
I just want the
Index.cshtml
view page to be reloaded with new data.I have debugged the code thoroughly. Infact, clicking on the"ul"
when the control goes to theIndex(string value)
method the model object is populated with new data and even in the cshtml page the Model is showing the new list in the debugger, but the view is NOT getting refreshed. I really don't know why.. Can anyone help me plz? If I have gone wrong horribly somewhere please excuse my ignorance as I am new to MVC. Thanks in advance... Controller:namespace MVCTestApp1.Controllers { public class TestController : Controller { // // GET: /Test/ public ActionResult Index() { ModelState.Clear(); List<string> ll = new List<string>(); ll.Add("qq"); ll.Add("aa"); ll.Add("zz"); return View(ll); } [HttpPost] public ActionResult Index(string value) { ModelState.Clear(); List<string> ll = new List<string>(); ll.Add("kkk"); ll.Add("qq"); ll.Add("aa"); ll.Add("zz"); return View(ll); } } } View: @model IEnumerable<string> @{ ViewBag.Title = "Index"; } @using (Html.BeginForm()) { @Html.ValidationSummary(true) <h2>Index</h2> <ul id="bb"> @foreach (var i in Model) { <li>@Html.DisplayFor(ir=>i)</li> } </ul> }