MVC4 C# - Want to save a image to a folder and saving the url in database
17,132
For file uploading, you can use this code. Image saved to folder and filename stored to database:
In controller:
[HttpPost]
public ActionResult Create(EventModel eventmodel, HttpPostedFileBase file)
{
if (ModelState.IsValid)
{
var filename = Path.GetFileName(file.FileName);
var path = Path.Combine(Server.MapPath("~/Uploads/Photo/"), filename);
file.SaveAs(path);
tyre.Url = filename;
_db.EventModels.AddObject(eventmodel);
_db.SaveChanges();
return RedirectToAction("Index");
}
return View(eventmodel);
}
And View:
<div>
Image
<input type="file" name="file" id="file" />
@Html.HiddenFor( model => model.ImageUrl)
@Html.ValidationMessageFor( model => model.Url )
</div>
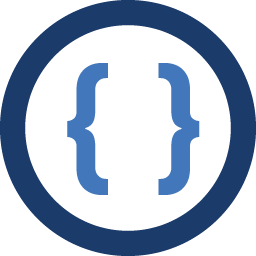
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm pretty new with MVC4 coding. Have been programming in SharePoint before.
The issue i have is that i want to save a image to a specific folder (Let say App_Data) and also save the url of the image to a string in a database. Would be great if anyone could help me with this issue.
The code i got right now is.
Models > ImageUpload.cs
public class ImageUpload { public int ID { get; set; } public string Title { get; set; } public string Url { get; set; } } public class ImageUploadDBContext : DbContext { public DbSet<ImageUpload> ImageUploads { get; set; } }
Controllers > ImageUploadController.cs
[HttpPost] [ValidateAntiForgeryToken] public ActionResult Create(ImageUpload imageupload) { if (ModelState.IsValid) { db.ImageUploads.Add(imageupload); db.SaveChanges(); return RedirectToAction("Index"); } return View(imageupload); } [HttpPost] public ActionResult Upload(HttpPostedFileBase[] files) { foreach (HttpPostedFileBase file in files) { string picture = Path.GetFileName(file.FileName); string path = Path.Combine(Server.MapPath("~/App_Data"), picture); string[] paths = path.Split('.'); string time = DateTime.UtcNow.ToString(); time = time.Replace(" ", "-"); time = time.Replace(":", "-"); file.SaveAs(paths[0] + "-" + time + ".jpg"); } ViewBag.Message = "File(s) uploaded successfully"; return RedirectToAction("Index"); }
View > ImageUpload > Index.cshtml
@{ ViewBag.Title = "Index"; } <h2>Index</h2> <p> @Html.ActionLink("Create New", "Create") </p> <table> <tr> <th> @Html.DisplayNameFor(model => model.Title) </th> <th> @Html.DisplayNameFor(model => model.Url) </th> <th> Preview </th> <th></th> </tr> @foreach (var item in Model) { <tr> <td> @Html.DisplayFor(modelItem => item.Title) </td> <td> @Html.DisplayFor(modelItem => item.Url) </td> <td> <img border="0" src="@Html.DisplayFor(modelItem => item.Url)" alt="@Html.DisplayFor(modelItem => item.Title)"> </td> <td> @Html.ActionLink("Edit", "Edit", new { id=item.ID }) | @Html.ActionLink("Details", "Details", new { id=item.ID }) | @Html.ActionLink("Delete", "Delete", new { id=item.ID }) </td> </tr> } </table>
View > ImageUpload > Create.cshtml
@{ ViewBag.Title = "Create"; } <h2>Create</h2> @using (Html.BeginForm()) { @Html.AntiForgeryToken() @Html.ValidationSummary(true) <fieldset> <legend>ImageUpload</legend> @using (Html.BeginForm()) { <div class="editor-label"> <b>@Html.LabelFor(Model => Model.Title)</b> </div> <div class="editor-field"> @Html.EditorFor(Model => Model.Title) @Html.ValidationMessageFor(Model => Model.Title) </div> <div class="editor-label"> <b>@Html.LabelFor(Model => Model.Url)</b> </div> <div> @Html.EditorFor(Model => Model.Url) <input type="file" name="files" value="" multiple="multiple"/> </div> <div> <input type="submit" value="Submit" /> </div> } </fieldset> } <div> @Html.ActionLink("Back to List", "Index") </div> @section Scripts { @Scripts.Render("~/bundles/jqueryval") }