MyBatis:collection via annotation in one query
Solution 1
AFAIK, you cannot use JOIN
s if you are using mapping with annotations.
From the doc, about the usage of @Many
,
A mapping to a collection property of a complex type. Attributes: select, which is the fully qualified name of a mapped statement (i.e. mapper method) that can load a collection of instances of the appropriate types, fetchType, which supersedes the global configuration parameter lazyLoadingEnabled for this mapping. NOTE You will notice that join mapping is not supported via the Annotations API. This is due to the limitation in Java Annotations that does not allow for circular references.
You can directly use your ResultMap with annotations if you like:
@Select("SELECT QUERY")
@ResultMap("readItemsRM")
public List<Item> select();
Solution 2
I found out that you can actually do one-to-many or one-to-one joins using Java annotations on MyBatis
public class Master {
private String nama;
private Short usia;
private List<Contoh> contohs;
}
public interface MasterMapper {
@Select("SELECT master.nama, master.usia FROM test.master WHERE master.nama = #{nama}")
@Results(value = {
@Result(property="nama", column="nama"),
@Result(property="usia", column="usia"),
@Result(property="contohs", javaType=List.class, column="nama",
many=@Many(select="getContohs"))
})
Master selectUsingAnnotations(String nama);
@Select("SELECT contoh.id, contoh.nama, contoh.alamat "
+ " FROM test.contoh WHERE contoh.nama = #{nama}")
List<Contoh> getContohs(String nama);
}
There are more details in this link
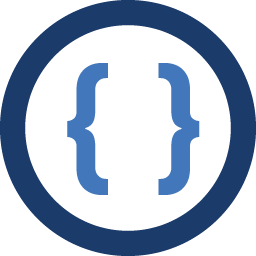
Admin
Updated on July 24, 2022Comments
-
Admin almost 2 years
I have an xml mapper - one select and one result mapper. It works without problems. But I want to use annotations. My mapper:
<resultMap id="readItemsRM" type="com.company.Item"> <id property="id" column="Id"/> <result property="comment" column="Comment"/> <collection property="textItems" ofType="com.company.TextItem"> <id property="id" column="TxId"/> <result property="name" column="TxName"/> </collection> </resultMap>
So I did like this
@Results({ @Result(id=true, property="id", column="Id"), @Result(property="comment", column="Comment"), ///,??? }) public List<Item> select();
I can't understand how can I map my collection via annotation without executing one more sql query. As all the examples I found suppose executing one more query. Please help.