Net Core API: Purpose of ProducesResponseType
Solution 1
I think it can come handy for non-success (200) return codes. Say if one of the failure status codes returns a model that describes the problem, you can specify that the status code in that case produces something different than the success case. You can read more about that and find examples here: https://docs.microsoft.com/en-us/aspnet/core/web-api/action-return-types?view=aspnetcore-2.2
Solution 2
Although the correct answer is already submitted, I would like to provide an example. Assume you have added the Swashbuckle.AspNetCore package to your project, and have used it in Startup.Configure(...) like this:
app.UseSwagger();
app.UseSwaggerUI(options =>
{
options.SwaggerEndpoint("/swagger/v1/swagger.json", "My Web Service API V1");
options.RoutePrefix = "api/docs";
});
Having a test controller action endpoint like this:
[HttpGet]
public ActionResult GetAllItems()
{
if ((new Random()).Next() % 2 == 0)
{
return Ok(new string[] { "value1", "value2" });
}
else
{
return Problem(detail: "No Items Found, Don't Try Again!");
}
}
Will result in a swagger UI card/section like this (Run the project and navigate to /api/docs/index.html):
As you can see, there is no 'metadata' provided for the endpoint.
Now, update the endpoint to this:
[HttpGet]
[ProducesResponseType(typeof(IEnumerable<string>), 200)]
[ProducesResponseType(404)]
public ActionResult GetAllItems()
{
if ((new Random()).Next() % 2 == 0)
{
return Ok(new string[] { "value1", "value2" });
}
else
{
return Problem(detail: "No Items Found, Don't Try Again!");
}
}
This won't change the behavior of your endpoint at all, but now the swagger page looks like this:
This is much nicer, because now the client can see what are the possible response status codes, and for each response status, what is the type/structure of the returned data. Please note that although I have not defined the return type for 404, but ASP.NET Core (I'm using .NET 5) is smart enough to set the return type to ProblemDetails.
If this is the path you want to take, it's a good idea to add the Web API Analyzer to your project, to receive some useful warnings.
p.s. I would also like to use options.DisplayOperationId(); in app.UseSwaggerUI(...) configuration. By doing so, swagger UI will display the name of the actual .NET method that is mapped to each endpoint. For example, the above endpoint is a GET to /api/sample but the actual .NET method is called GetAllItems()
Solution 3
It's for producing Open API metadata for API exploration/visualization tools such as Swagger (https://swagger.io/), to indicate in the documentation what the controller may return.
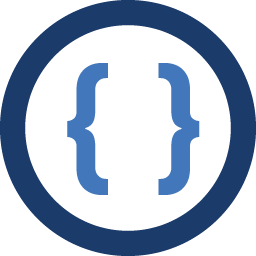
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
I want to understand the purpose of
ProducesResponseType.
Microsoft defines as
a filter that specifies the type of the value and status code returned by the action.
So I am curious what are consequences if
- a person does not place ProductResponseType?
- How is system at a disadvantage, or is there negative consequence?
- Doesn't Microsoft API already automatically inherently know the type/value of status code returned?
[ProducesResponseType(typeof(DepartmentDto), StatusCodes.Status200OK)] [ProducesResponseType(StatusCodes.Status404NotFound)]
Documentation from the Microsoft: ProducesResponseTypeAttribute Class
-
Andy about 4 yearsquote from that linked page "This attribute produces more descriptive response details for web API help pages generated by tools like Swagger." so I guess it's for documentation purposes (and possibly could be used by static code analysis).
-
user1172763 over 3 yearsLooks like more cruft. If boilerplate XML comments didn't clutter up your code enough for your liking, now there's this. Boy, it sure looks smart in the text editor, though!
-
Patrick Szalapski over 2 yearsFor controllers that return a ordinary type rather than an ActionResult, could/should there a be way to have Swagger automatically infer that the C# return type is for the success/200 and thus not have to add these redundant
[ProducesResponseType(typeof(...), 200)]
attributes on every controller method? -
Siavash Mortazavi over 2 years@PatrickSzalapski I believe you can use compile-time code injectors, like github.com/Fody/Fody to avoid bloating your code with repeated attributes. I'm almost certain there are some Roslyn compilation services / code generation features, capable of achieving something similar, e.g. mark the controller class and action method as 'partial', then generate a partial class for it with partial method declarations that are decorated with all the required attributes. (I have not done this myself, just thinking aloud! LOL)