.net core IActionResult return OK()
12,019
I found what was the problem.
In Controller I add one more method
[HttpGet("GetMachines")] public IActionResult GetMachines() { try { var results = _repository.GetAllMachineTypes(); return Ok(Mapper.Map<IEnumerable<MachineTypeViewModel>>(results)); } catch (Exception ex) { _logger.LogError($"Failed to get all Machine types: {ex}"); return BadRequest("Error Occurred"); } }
Second I changed machineController.js
(function () { "use strict"; angular.module("app") .controller("machineController", machineController); function machineController($http) { /* jshint validthis:true */ var vm = this; vm.machines = []; vm.errorMessage = ""; $http.get("/machines/GetMachines") .then(function (response) { // Success angular.copy(response.data, vm.machines); }, function (error) { // Failure vm.errorMessage = "Failed: " + error; }); } })();
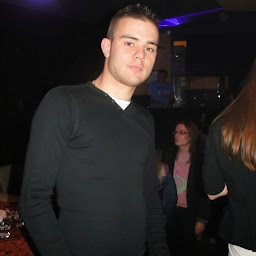
Author by
Danijel Boksan
Updated on June 07, 2022Comments
-
Danijel Boksan almost 2 years
I have follow next example Link to source
And when I create my controller(which should be same as his TripsController) to return Ok with data, browser is not parsing to HTML it is only shown json format in browser.
using System; using System.Collections.Generic; using AutoMapper; using Microsoft.AspNetCore.Authorization; using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Logging; using RnD.Models.Repository; using RnD.Models.ViewModels; namespace RnD.Controllers.Web { [Route("/machines")] [Authorize] public class MachineTypeController : Controller { private ILogger<MachineTypeController> _logger; private IMachineTypeRepository _repository; public MachineTypeController(IMachineTypeRepository repository, ILogger<MachineTypeController> logger) { _logger = logger; _repository = repository; } [HttpGet("")] public IActionResult Index() { try { var results = _repository.GetAllMachineTypes(); return Ok(Mapper.Map<IEnumerable<MachineTypeViewModel>>(results)); } catch (Exception ex) { _logger.LogError($"Failed to get all Machine types: {ex}"); return BadRequest("Error Occurred"); } } } }
If I put to return View it will work correctly.
Here is Startup.cs
using System.Threading.Tasks; using AutoMapper; using Microsoft.AspNetCore.Authentication.Cookies; using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.AspNetCore.Identity.EntityFrameworkCore; using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Logging; using Newtonsoft.Json.Serialization; using RnD.Models.DataFixtures; using RnD.Models.Entity; using RnD.Models.Repository; using RnD.Models.ViewModels; namespace RnD { public class Startup { private IHostingEnvironment _env; private IConfigurationRoot _config; public Startup(IHostingEnvironment env) { _env = env; var builder = new ConfigurationBuilder() .SetBasePath(_env.ContentRootPath) .AddJsonFile("config.json") .AddEnvironmentVariables(); _config = builder.Build(); } public void ConfigureServices(IServiceCollection services) { services.AddSingleton(_config); services.AddDbContext<RnDContext>(); services.AddIdentity<ApplicationUser, IdentityRole>(config => { config.User.RequireUniqueEmail = false; config.Password.RequireDigit = false; config.Password.RequireUppercase = false; config.Password.RequiredLength = 8; config.Cookies.ApplicationCookie.LoginPath = "/auth/login"; config.Cookies.ApplicationCookie.Events = new CookieAuthenticationEvents() { OnRedirectToLogin = async ctx => { if (ctx.Request.Path.StartsWithSegments("/api") && ctx.Response.StatusCode == 200) { ctx.Response.StatusCode = 401; } else { ctx.Response.Redirect(ctx.RedirectUri); } await Task.Yield(); } }; }).AddEntityFrameworkStores<RnDContext>(); services.AddScoped<IMachineTypeRepository, MachineTypeRepository>(); services.AddTransient<RnDContextSeedData>(); services.AddLogging(); services.AddMvc(config => { if (_env.IsProduction()) { config.Filters.Add(new RequireHttpsAttribute()); } }) .AddJsonOptions(config => { config.SerializerSettings.ContractResolver = new CamelCasePropertyNamesContractResolver(); }); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IHostingEnvironment env, RnDContextSeedData seeder, ILoggerFactory loggerFactory ) { Mapper.Initialize(config => { config.CreateMap<MachineTypeViewModel, MachineType>().ReverseMap(); }); loggerFactory.AddConsole(); if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); loggerFactory.AddDebug(LogLevel.Information); } else { loggerFactory.AddDebug(LogLevel.Error); } app.UseStaticFiles(); app.UseIdentity(); app.UseMvc(config => { config.MapRoute( name: "Default", template: "{controller}/{action}/{id?}", defaults: new { controller = "Home", action = "Index" } ); }); seeder.EnsureSeedData().Wait(); } } }
Here is code from angular
app.js
// app.js (function () { "use strict"; angular.module("app",[]); })();
machineController.js
// machineController.js (function () { "use strict"; angular.module("app") .controller("machineController", machineController); function machineController($http) { /* jshint validthis:true */ var vm = this; vm.machines = []; vm.errorMessage = ""; $http.get("/machines") .then(function (response) { // Success angular.copy(response.data, vm.machines); }, function (error) { // Failure vm.errorMessage = "Failed: " + error; }); } })();
Index.cshtml
@model IEnumerable<RnD.Models.Entity.MachineType> @{ ViewBag.Title = "Machine Type List"; } @section scripts{ <script src="~/lib/angular/angular.js"></script> <script src="~/js/app.js"></script> <script src="~/js/machineController.js"></script> } <div class="row" ng-app="app"> <div ng-controller="machineController as vm" class="col-md-6 col-md-offset-6"> <table class="table table-responsive"> <tr ng-repeat="machine in vm.machines"> <td>{{machine.name}}</td> </tr> </table> </div> </div>