.NET: Get all Outlook calendar items
Solution 1
I believe that you must Restrict or Find in order to get recurring appointments, otherwise Outlook won't expand them. Also, you must Sort by Start before setting IncludeRecurrences.
Solution 2
I wrote similar code, but then found the export functionality:
Application outlook;
NameSpace OutlookNS;
outlook = new ApplicationClass();
OutlookNS = outlook.GetNamespace("MAPI");
MAPIFolder f = OutlookNS.GetDefaultFolder(OlDefaultFolders.olFolderCalendar);
CalendarSharing cs = f.GetCalendarExporter();
cs.CalendarDetail = OlCalendarDetail.olFullDetails;
cs.StartDate = new DateTime(2011, 11, 1);
cs.EndDate = new DateTime(2011, 12, 31);
cs.SaveAsICal("c:\\temp\\cal.ics");
Solution 3
LinqPad snipped that works for me:
//using Microsoft.Office.Interop.Outlook
Application a = new Application();
Items i = a.Session.GetDefaultFolder(OlDefaultFolders.olFolderCalendar).Items;
i.IncludeRecurrences = true;
i.Sort("[Start]");
i = i.Restrict(
"[Start] >= '10/1/2013 12:00 AM' AND [End] < '10/3/2013 12:00 AM'");
var r =
from ai in i.Cast<AppointmentItem>()
select new {
ai.Categories,
ai.Start,
ai.Duration
};
r.Dump();
Solution 4
If you need want to access the shared folder from your friend, then you can set your friend as the recipient. Requirement: his calendar must be shared first.
// Set recepient
Outlook.Recipient oRecip = (Outlook.Recipient)oNS.CreateRecipient("[email protected]");
// Get calendar folder
Outlook.MAPIFolder oCalendar = oNS.GetSharedDefaultFolder(oRecip, Outlook.OlDefaultFolders.olFolderCalendar);
Solution 5
There is no need to expand recurring items manually. Just ensure you sort the items before using IncludeRecurrences.
Here is VBA example:
tdystart = VBA.Format(#8/1/2012#, "Short Date")
tdyend = VBA.Format(#8/31/2012#, "Short Date")
Dim folder As MAPIFolder
Set appointments = folder.Items
appointments.Sort "[Start]" ' <-- !!! Sort is a MUST
appointments.IncludeRecurrences = True ' <-- This will expand reccurent items
Set app = appointments.Find("[Start] >= """ & tdystart & """ and [Start] <= """ & tdyend & """")
While TypeName(app) <> "Nothing"
MsgBox app.Start & " " & app.Subject
Set app = appointments.FindNext
Wend
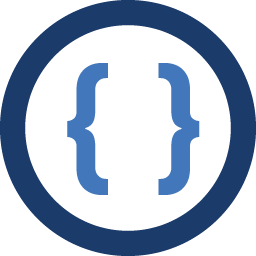
Admin
Updated on June 10, 2020Comments
-
Admin almost 4 years
How can I get all items from a specific calendar (for a specific date). Lets say for instance that I have a calendar with a recurring item every Monday evening. When I request all items like this:
CalendarItems = CalendarFolder.Items; CalendarItems.IncludeRecurrences = true;
I only get 1 item...
Is there an easy way to get all items (main item + derived items) from a calendar? In my specific situation it can be possible to set a date limit but it would be cool just to get all items (my recurring items are time limited themselves).
I'm using the Microsoft Outlook 12 Object library (Microsoft.Office.Interop.Outlook).