Next.js: How to dynamically import external client-side only React components into Server-Side-Rendering apps developed?
Solution 1
Next js now has its own way of doing dynamic imports with no SSR.
import dynamic from 'next/dynamic'
const DynamicComponentWithNoSSR = dynamic(
() => import('../components/hello3'),
{ ssr: false }
)
Here is the link of their docs: next js
Solution 2
I've managed to resolve this by first declaring a variable at the top:
let Chat = ''
then doing the import this way in componentDidMount:
async componentDidMount(){
let result = await import('react-chat-popup')
Chat = result.Chat
this.setState({
appIsMounted: true
})
}
and finally render it like this:
<NoSSR>
{this.state.appIsMounted? <Chat/> : null}
</NoSSR>
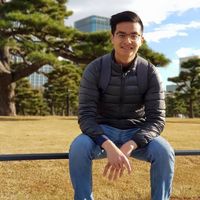
Lew Wei Hao
Updated on June 04, 2022Comments
-
Lew Wei Hao 7 months
I know this question has been asked multiple times before but none of the solution seems to work.
I'm trying to use the library 'react-chat-popup' which only renders on client side in a SSR app.(built using next.js framework) The normal way to use this library is to call
import {Chat} from 'react-chat-popup'
and then render it directly as<Chat/>
.The solution I have found for SSR apps is to check if
typedef of window !=== 'undefined'
in thecomponentDidMount
method before dynamically importing the library as importing the library normally alone would already cause thewindow is not defined
error. So I found the link https://github.com/zeit/next.js/issues/2940 which suggested the following:Chat = dynamic(import('react-chat-popup').then(m => { const {Foo} = m; Foo.__webpackChunkName = m.__webpackChunkName; return Foo; }));
However, my foo object becomes null when I do this. When I print out the m object in the callback, i get
{"__webpackChunkName":"react_chat_popup_6445a148970fe64a2d707d15c41abb03"}
How do I properly import the library and start using the<Chat/>
element in this case?