NFC intent -> get info from the tag
Solution 1
You should first call resolveIntent
method.
You fire an intent for discovered that should be resolved.
Resolving it contains getting Tag data by calling getParcelableExtra()
method on intent.
That will give you parcelable which you assign to a tag.
If you want code then post a comment here.
Solution 2
I have created an Android utility project which parses NDEF messages into higher-level objects. The same site includes abstract activity templates (and demo) for doing what you are looking to do (i.e. in foreground mode).
I've also created a graphical NDEF editor you might be intersted in.
Edit: Linked code is much improved
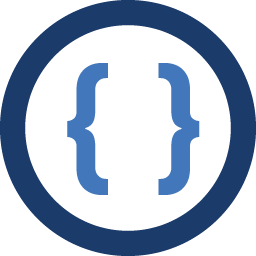
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm struggling with NFC and intents, I can discover tags, but how could I read info out from them?
As the code reveals: I get Discovered tag with intent: Intent { act= android.nfc.action TECH_DISCOVERED flg=0x13400000 cmp=com.example.android.apid/.nfc. Techfilter(has extra)}
I want to get info from the tag, should I be parsing the bytes etc?
Best Regs h.
/* * Copyright (C) 2011 The Android Open Source Project * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License */ package com.example.android.apis.nfc; import java.util.List; import com.example.android.apis.R; import android.app.Activity; import android.app.PendingIntent; import android.content.Intent; import android.content.IntentFilter; import android.content.IntentFilter.MalformedMimeTypeException; import android.nfc.NfcAdapter; import android.nfc.Tag; import android.nfc.tech.NfcF; import android.os.Bundle; import android.util.Log; import android.widget.TextView; /** * An example of how to use the NFC foreground dispatch APIs. This will intercept any MIME data * based NDEF dispatch as well as all dispatched for NfcF tags. */ public class ForegroundDispatch extends Activity { //StringBuilder detailsRead = new StringBuilder(); private NfcAdapter mAdapter; private PendingIntent mPendingIntent; private IntentFilter[] mFilters; private String[][] mTechLists; private TextView mText; private int mCount = 0; static final String TAG = "TagReadActivity"; @Override public void onCreate(Bundle savedState) { super.onCreate(savedState); setContentView(R.layout.foreground_dispatch); mText = (TextView) findViewById(R.id.text); //resolveIntent(getIntent()); mText.setText("Scan a tag"); mAdapter = NfcAdapter.getDefaultAdapter(this); // Create a generic PendingIntent that will be deliver to this activity. The NFC stack // will fill in the intent with the details of the discovered tag before delivering to // this activity. mPendingIntent = PendingIntent.getActivity(this, 0, new Intent(this, getClass()).addFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP), 0); // Setup an intent filter for all MIME based dispatches IntentFilter ndef = new IntentFilter(NfcAdapter.ACTION_TAG_DISCOVERED); try { ndef.addDataType("*/*"); } catch (MalformedMimeTypeException e) { throw new RuntimeException("fail", e); } mFilters = new IntentFilter[] { ndef, }; // Setup a tech list for all NfcF tags mTechLists = new String[][] { new String[] { NfcF.class.getName() } }; } /** void resolveIntent(Intent intent) { // TODO Auto-generated method stub String action = intent.getAction(); if (NfcAdapter.ACTION_TAG_DISCOVERED.equals(action)) { // When a tag is discovered we send it to the service to be save. We // include a PendingIntent for the service to call back onto. This // will cause this activity to be restarted with onNewIntent(). At // that time we read it from the database and view it. Parcelable[] rawMsgs = intent.getParcelableArrayExtra(NfcAdapter.EXTRA_NDEF_MESSAGES); NdefMessage[] msgs; if (rawMsgs != null) { msgs = new NdefMessage[rawMsgs.length]; for (int i = 0; i < rawMsgs.length; i++) { msgs[i] = (NdefMessage) rawMsgs[i]; } } else { // Unknown tag type byte[] empty = new byte[] {}; NdefRecord record = new NdefRecord(NdefRecord.TNF_UNKNOWN, empty, empty, empty); NdefMessage msg = new NdefMessage(new NdefRecord[] {record}); msgs = new NdefMessage[] {msg}; } // Setup the views } else { Log.e(TAG, "Unknown intent " + intent); mText.setText("My thing going on " + ++mCount + " with intent: " + action); finish(); return; } } **/ @Override public void onResume() { super.onResume(); mAdapter.enableForegroundDispatch(this, mPendingIntent, mFilters, mTechLists); } @Override public void onNewIntent(Intent intent) { Log.i("Foreground dispatch", "Discovered tag with intent: " + intent); mText.setText("Discovered tag " + ++mCount + " with intent: " + intent); } @Override public void onPause() { super.onPause(); mAdapter.disableForegroundDispatch(this); } }