Node + Express Session Expiration?
Solution 1
You can use expires attribute instead of maxAge. It takes Date object as value. Also, check session cookie exipres on client after they set. Maybe session ended by server (i.e. memcached restart).
Code example:
app.use(express.session(
{ secret: "secret", store: new MemoryStore(), expires: new Date(Date.now() + (30 * 86400 * 1000))
}));
but
app.use(express.session(
{ secret: "secret", store: new MemoryStore(), maxAge: Date.now() + (30 * 86400 * 1000)
}));
works fine for me too.
Solution 2
The accepted answer by @Vadim Baryshev is flawed in at least the most recent express-session implementations. When your app starts up you create the session implementation and set the max age. As for the answer using expires, that is not recommended, stick with maxAge.
This means that the maxAge example as written would expire every single session a month after the server starts, and every session created in the future would be expired immediately. Until the server was restarted, there would not be any valid sessions.
Instead of passing a date object, just pass the number of milliseconds to the maxAge property like so:
app.use(
session({
...,
cookie: {
maxAge: 30 * 24 * 60 * 60 * 1000
}
})
);
Solution 3
maxAge
means how long the session lasts, in ms;
expires
means when the session gonna expire, ie: a date object
var hour = 3600000
req.session.cookie.expires = new Date(Date.now() + hour)
or
var hour = 3600000
req.session.cookie.maxAge = hour
Solution 4
The documentation does not recommend setting cookie.expires directly. Instead, set cookie.maxAge directly.
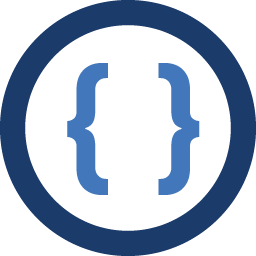
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I have an app in express and I have a login form. I need sessions to last for 1 month do I set maxAge to a month in milliseconds.
I left two computers on and logged in for 24 hours and when I came back both were logged out.
How do I fix this/achieve what I'm trying to do? Thanks.
-
Admin almost 12 yearsCould you give me an example of how to use the expires attribute?
-
Vadim Baryshev almost 12 yearsapp.use(express.session({ secret: "secret", store: new MemoryStore(), expires: new Date(Date.now() + (30 * 86400 * 1000)) })); but app.use(express.session({ secret: "secret", store: new MemoryStore(), maxAge: Date.now() + (30 * 86400 * 1000) })); works fine for me too.
-
rdrey almost 12 yearsthe
MemoryStore
only stores sessions for as long as the express App is running. So if you restart your server at any point, the cookies aren't linked to an in-memory session any more and express will make your users sign in again. -
Stevie over 10 yearsMaybe I misunderstand how this works, but ... the "expires" example will set an expiry date relative to when the app is started, so one month after the app starts all sessions will immediately expire (incl. any new sessions created after this point)? and the maxAge example sets an age which is (current-offset-from-epoch + 30 days in millis) which is about 44 years from when the app starts ... shouldn't they be wrapped in functions or something?
-
Janac Meena almost 6 yearsThe docs suggest not to use expires, and to prefer using maxAge: npmjs.com/package/express-session#cookieexpires
-
Daniel Carpio Contreras over 5 yearsWhy do you set "maxAge: Date.now() + (30 * 86400 * 1000)" in your example? I mean, why the expression "Date.now()" at start? According to docs npmjs.com/package/express-session#cookiemaxage It says "This is done by taking the current server time and adding maxAge milliseconds to the value to calculate an Expires datetime."
-
MartyBoggs almost 5 yearsDaniel's right. The maxAge property should not include a Date.now() component.
-
krivar over 4 yearsYou're mixing up maxAge and expires again. 6 years old answer, still incorrect :D Read Dong's answer: stackoverflow.com/a/30562724/1905229
-
Sgnl almost 4 yearsdownvoted - expires is not recommended to be used and using
Date.now()
is not correct