Nodejs - Req.body undefined in post with express 4.9.0
Solution 1
By default cURL uses Content-Type: application/x-www-form-urlencoded
for form submissions that do not contain files.
For urlencoded forms your data needs to be in the right format: curl -X POST -d 'foo=bar&baz=bla' http://127.0.0.1:3000/module
or curl -X POST -d 'foo=bar' -d 'baz=bla' http://127.0.0.1:3000/module
.
For JSON, you'd have to explicitly set the right Content-Type
: curl -H "Content-Type: application/json" -d '{"foo":"bar","baz":"bla"}' http://127.0.0.1:3000/module
.
Also as @Brett noted, you need to app.use()
your middleware before that POST route somewhere (outside of a route handler).
Solution 2
You are placing the express configuration for body-parser
in the wrong location.
var app = require('express')();
var bodyParser = require('body-parser');
var multer = require('multer');
// these statements config express to use these modules, and only need to be run once
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(multer);
// set up your routes
app.get('/', function(req, res) {
res.send("Hello world!\n");
});
app.post('/module', function(req, res) {
console.log(req);
console.log(req.body);
});
app.listen(3000);
module.exports = app;
Solution 3
You must make sure that you define all express configurations before defining routes. as body-parser is responsible for parsing requst's body.
var express = require('express'),
app = express(),
port = parseInt(process.env.PORT, 10) || 8080;
//you can remove the app.configure at all in case of it is not supported
//directly call the inner code
app.configure(function(){
app.use(bodyParser.urlencoded());
//in case you are sending json objects
app.use(bodyParser.json());
app.use(app.router);
});
app.listen(port);
app.post("/module", function(req, res) {
res.send(req.body);
});
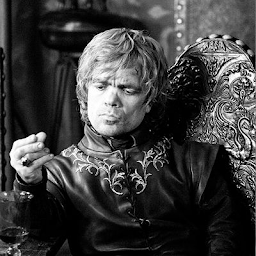
Tyrion
Updated on June 16, 2022Comments
-
Tyrion almost 2 years
I'm a beginner of nodejs, I try to know req.body using a middleware body-parse or using nothing, but both found req.body is undefined. Here is my code
var app = require('express')(); var bodyParser = require('body-parser'); var multer = require('multer'); app.get('/', function(req, res) { res.send("Hello world!\n"); }); app.post('/module', function(req, res) { console.log(req); app.use(bodyParser.json()); app.use(bodyParser.urlencoded({ extended: true })); app.use(multer); console.log(req.body); }); app.listen(3000); module.exports = app;
And I use command
curl -X POST -d 'test case' http://127.0.0.1:3000/module
to test it.express's version: 4.9.0
node's version: v0.10.33Please help, thank you.
-
Tyrion over 9 yearsThank you for your help, I tried as you told me, but noting print to the terminator, I don't know why.
-
Tyrion over 9 yearsThank you for your help, I found code error, app.use(multer()).
-
mako almost 9 yearsThere is no app.configure in express 4.9