NotAMockException while Mockito.verify(Object,VerificationMode.atleast(2))
Solution 1
Well, that's exactly what mockito says, you are not passing a mock to verify !
ConsumerImpl<String> consumer = new ConsumerImpl<String>(mConsumer, parserTest);
String mes=Mockito.verify(consumer,VerificationModeFactory.times(3)).consumeMessage(10,TimeUnit.SECONDS);
Plus if you verified a mock why would you want to store the result of the invocation you verify, it wouldn't make sense since the consumer is mocked. Verify is to verify calls on mocked objects that are the collaborators of your unit tested object. Which in your case is not really clear.
Also you never use your mock mConsumer
instance.
You should definitely separate your test in 3 phase, one for the fixture, one for the action, and one for the verifications. Use the BDD terminology to achieve that, it augments understanding and readability for the tester and future reader of this code (And Mockito offers them in the API through BDDMockito).
As I don't really get what the code is trying to test from the code you gave, I'll be imagining things. So for example you'll write this kind of code (using import static
) :
// given a consumer
MessageConsumer message_consumer = mock(MessageConsumer.class);
String the_message_data = "new Message for Testing";
given(message_consumer.next(10, SECONDS)).willReturn(new Message(the_message_data.getBytes()));
// when calling the client of the customer (which is the unit that is tested)
new MessageProcessor(message_consumer).processAll();
// then verify that consumeMessage is called 3 times
verify(message_consumer, times(3)).consumeMessage(10, SECONDS);
Remember Mockito helps you focus on interactions between objects — as it's the most important notion of object oriented programming — and especially between the tested one and his collaborators that will certainly be mocked.
Solution 2
Usually we mock using @InjectMock and we try to verify a method called from inside the test case method. Here is one scenario which generally give issue.
public class A{
@Autowired
Service s
public void method1(){
method2();
}
public void method2(){
s.someMethod();
}
}
public class ATest{
@InjectMocks
A a;
public void testM1(){
a.method1();
Mockito.verify(a, Mockito.times(1)).method2();
}
}
This will always give "NoAMockException while Mockito.verify" instead of that we should use following verification.
public class ATest{
@InjectMocks
A a;
@Mock
Service s
public void testM1(){
a.method1();
Mockito.verify(s, Mockito.times(1)).someMethod();
}
}
Or if we want to verify() method2() then we have to @Mock class A instead of @InjectMock
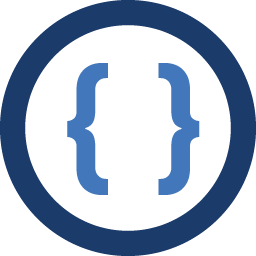
Admin
Updated on September 23, 2020Comments
-
Admin almost 4 years
I am using mockito for mock the unit test cases and am getting the following exception
org.mockito.exceptions.misusing.NotAMockException: Argument passed to verify() is of type ConsumerImpl and is not a mock! Make sure you place the parenthesis correctly! See the examples of correct verifications: verify(mock).someMethod(); verify(mock, times(10)).someMethod(); verify(mock, atLeastOnce()).someMetenter code herehod();
and my code is
MessageConsumer mConsumer = Mockito.mock(MessageConsumer.class); String data = "new Message for Testing"; Message message = new Message(data.getBytes()); Mockito.when(mConsumer.next(10, TimeUnit.SECONDS)).thenReturn(message); StringParserTest parserTest = new StringParserTest(); ConsumerImpl<String> consumer = new ConsumerImpl<String>(mConsumer, parserTest); String mes=Mockito.verify(consumer,VerificationModeFactory.times(3)).consumeMessage(10,TimeUnit.SECONDS);
Please some one help me to solve this problem
Thanks in Advance
SRN