NSDictionary keys sorted by value numerically
22,693
Solution 1
how about using keysSortedByValueUsingSelector (NSDictionary)
Seems to be what you need as per the documentation in XCode
Solution 2
NSString *defaultNames[] = {@"Matt", @"Terry",@"Jessica",@"Sean",nil};
NSNumber *defaultScores[] = {@"600", @"500",@"100",@"50", nil};
NSDictionary *newScoreDict = [NSDictionary dictionaryWithObjects:(id *)defaultScores forKeys:(id *)defaultNames count:7];
NSArray *currScores = [scoreDict keysSortedByValueUsingSelector:@selector(localizedStandardCompare:)];
Solution 3
@implementation NSString (numericComparison)
- (NSComparisonResult) floatCompare:(NSString *) other
{
float myValue = [self floatValue];
float otherValue = [other floatValue];
if (myValue == otherValue) return NSOrderedSame;
return (myValue < otherValue ? NSOrderedAscending : NSOrderedDescending);
}
- (NSComparisonResult) intCompare:(NSString *) other
{
int myValue = [self intValue];
int otherValue = [other intValue];
if (myValue == otherValue) return NSOrderedSame;
return (myValue < otherValue ? NSOrderedAscending : NSOrderedDescending);
}
@end
NSString *defaultNames[] = {@"Matt", @"Terry",@"Jessica",@"Sean",nil};
// NSNumber *defaultScores[] = {@"600", @"500",@"100",@"50", nil};
NSNumber *defaultScores[] = {
[NSNumber numberWithInt:600],
[NSNumber numberWithInt:500],
[NSNumber numberWithInt:100],
[NSNumber numberWithInt:50],
nil
};
NSDictionary *newScoreDict = [NSDictionary dictionaryWithObjects:(id *)defaultScores forKeys:(id *)defaultNames count:4];
NSArray *currScores = [newScoreDict keysSortedByValueUsingSelector:@selector(intCompare:NotSureWhatGoesHere:)];
I am still confused with the previous line ?
Do I just use
//
NSArray *currScores = [newScoreDict keysSortedByValueUsingSelector:@selector(intCompare:other:)];
//
Is the array of numbers OK, or is there an easier way ?
Thank You Very Much...
Solution 4
-compare: is a string compare. Pass a different method for the comparison, e.g:
@implementation NSString (numericComparison)
- (NSComparisonResult) compareNumerically:(NSString *) other
{
float myValue = [self floatValue];
float otherValue = [other floatValue];
if (myValue == otherValue) return NSOrderedSame;
return (myValue < otherValue ? NSOrderedAscending : NSOrderedDescending);
}
@end
In your specific case, you could use -intValue instead.
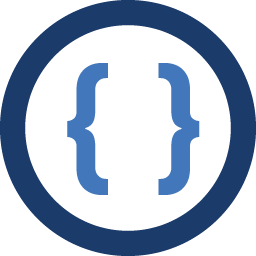
Author by
Admin
Updated on May 30, 2020Comments
-
Admin almost 4 years
I store names as keys and scores as values into an
NSDictionary
for saving inNSUserDefaults
. I then want to get back the keys sorted by score, but I can't seem to sort them numerically, only by string. The list of scores 100, 50, 300, 200, 500, for example, gives me 100, 200, 300, 50, 500.Can this be done or do I need to go about this differently?
NSString *defaultNames[] = {@"Matt", @"Terry",@"Jessica",@"Sean",nil}; NSNumber *defaultScores[] = {@"600", @"500",@"100",@"50", nil}; NSDictionary *newScoreDict = [NSDictionary dictionaryWithObjects:(id *)defaultScores forKeys:(id *)defaultNames count:7]; NSArray *currScores = [scoreDict keysSortedByValueUsingSelector:@selector(compare:)];