NSMutableURLRequest timeout interval not taken into consideration for POST requests
Solution 1
iOS 6 has fixed this issue.
NSMutableURLRequest *request = [NSMutableURLRequest
requestWithURL:[NSURL URLWithString:url]
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData timeoutInterval:20];
[request setHTTPMethod:method];
[request setHTTPBody:requestBody];
NSLog(@"%f", [request timeoutInterval]);
//20.0 in iOS 6
//240.0 in iOS 4.3, 5.0, 5.1
Solution 2
Fixed with Clay Chambers's suggestion: with a custom timer
Added a timer in a subclass of NSURLConnection
if (needsSeparateTimeout){
SEL sel = @selector(customCancel);
NSMethodSignature* sig = [self methodSignatureForSelector:sel];
NSInvocation* invocation = [NSInvocation invocationWithMethodSignature:sig];
[invocation setTarget:self];
[invocation setSelector:sel];
NSTimer *timer = [NSTimer timerWithTimeInterval:WS_REQUEST_TIMEOUT_INTERVAL invocation:invocation repeats:NO];
[[NSRunLoop mainRunLoop] addTimer:timer forMode:NSDefaultRunLoopMode];
}
In the custom cancel method the connection is cancelled
[super cancel];
Solution 3
It looks like problem described here is still facing iOS 7.1 (or reappeared). Fortunately it looks like setting timeoutIntervalForResource property on the configuration on the NSURLSession
can fix this.
EDIT
According to @XiOS observations this works for timeouts shorter than (around) 2 minutes.
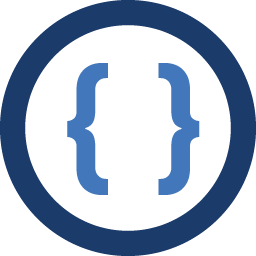
Admin
Updated on January 16, 2020Comments
-
Admin over 4 years
I have the following problem. On a
NSMutableURLRequest
using theHTTP
methodPOST
the timeout interval set for the connection is ignored. If the internet connection has a problem (wrong proxy, bad dns) the url request fails after about 2-4 minutes but not withNSLocalizedDescription = "timed out";
NSUnderlyingError = Error Domain=kCFErrorDomainCFNetwork Code=-1001 UserInfo=0x139580 "The request timed out.
If the
http
method used isGET
it works fine. The connection isasync
overhttps
.NSURL *url = [NSURL URLWithString:urlString]; NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url]; [request setTimeoutInterval:10]; //Set the request method to post [request setHTTPMethod:@"POST"]; if (body != nil) { [request setHTTPBody:body]; } // Add general parameters to the request if (authorization){ [request addValue: authorization forHTTPHeaderField:@"Authorization"]; } [request addValue: WS_HOST forHTTPHeaderField:@"Host"]; [request addValue:@"text/xml" forHTTPHeaderField:@"Content-Type"]; [[NSURLCache sharedURLCache] setDiskCapacity:0]; [self addToQueueRequest:request withDelegate:delegate]; '