Object id in Django url
13,764
Solution 1
Simply
url('^post/(?P<post_id>\d+)/$',Dashviews.public_post_view, name='public_post_view'),
in views.py
:
def public_post_view(request, post_id):
# do some stuff
in templates:
{% url 'public_post_view' post.id %}
Solution 2
A better way to that is by adding a get_absolute_url to the model:
First, define your view for the model:
def public_post_view(request, post_id):
# print(post_id) or whatever you want
Then create the url to map that view:
urls.py
url('^post/(?P<post_id>\d+)/$', Dashviews.public_post_view, name='public_post_view'),
Then, make every object in your Post model able to create its own url.
models.py
from django.core.urlresolvers import reverse_lazy
class Post(models.Model):
user = models.ForeignKey(User)
posted = models.DateTimeField(auto_now_add=True)
content = models.CharField(max_length=150)
picturefile = models.ImageField(upload_to="post_content", blank=True)
def get_absolute_url(self):
return reverse_lazy('public_post_view', kwargs={'post_id': self.id})
Now you're able to just use
<a href="{{ post.get_absolute_url }}">Go to the post</a>
in your templates. So whenever you're displaying something like {{ post.content }}
or stuff like that, you can also use the get_absolute_url method to get its URL.
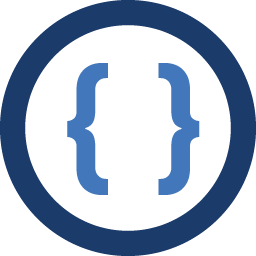
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have a Post model like the one below:
class Post(models.Model): user = models.ForeignKey(User) posted = models.DateTimeField(auto_now_add=True) content = models.CharField(max_length=150) picturefile = models.ImageField(upload_to="post_content", blank=True)
I want to be able to put the id of each post in an url so I can visit each post individually. I did this before with the id of a user and was able to view the user's profile page.
url(r'^profile/(?P<username>\w+)/$', Dashviews.public_profile_view, name='public_profile_view'),
But how do I make the same type of url, with an id of a post?
-
Admin about 7 yearsthis returns: the view got an unexpected keyword argument 'post_id'
-
Satevg about 7 years@Acework I guess you need to define your function as def public_post_view(request, post_id)