Obtaining reference to Class instance by string name - VB.NET
I don´t know if I´ve understood you well, but my answer is yes, you can do it by reflection. You´ll need to import System.Reflection
namespace.
Here is an example:
' Note that I´m in namespace ConsoleApplication1
Dim NameOfMyClass As String = "ConsoleApplication1.MyClassA"
Dim NameOfMyPropertyInMyClass As String = "MyFieldInClassA"
' Note that you are getting a NEW instance of MyClassA
Dim MyInstance As Object = Activator.CreateInstance(Type.GetType(NameOfMyClass))
' A PropertyInfo object will give you access to the value of your desired field
Dim MyProperty As PropertyInfo = MyInstance.GetType().GetProperty(NameOfMyPropertyInMyClass)
Once you have MyProperty, uou can get the value of your property, just like this:
MyProperty.GetValue(MyInstance, Nothing)
Passing to the method the instace of what you want to know the value.
Tell me if this resolve your question, please :-)
EDIT
This would be ClassA.vb
Public Class MyClassA
Private _myFieldInClassA As String
Public Property MyFieldInClassA() As String
Get
Return _myFieldInClassA
End Get
Set(ByVal value As String)
_myFieldInClassA = value
End Set
End Property
End Class
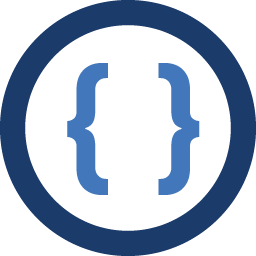
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Is it possible using Reflection or some other method to obtain a reference to a specific class instance from the name of that class instance?
For example the framework for the applications i develop heavily uses public class instances such as: Public bMyreference as MyReference = new MyReference
Then throughout the application bMyReference is used by custom controls and code.
One of the properties of the custom controls is the "FieldName" which references a Property in these class instances (bMyReference.MyField) as a string.
What i would like to be able to do is analyze this string "bMyReference.MyField" and then refer back to the actual Instance/Property.
In VB6 I would use an EVAL or something simular to convert the string to an actual object but this obviously doesn't work in VB.net
What I'm picturing is something like this
Dim FieldName as String = MyControl.FieldName ' sets FielName to bMyReference.MyField Dim FieldObject() as String = FieldName.Split(".") ' Split into the Object / Property Dim myInstance as Object = ......... ' Obtain a reference to the Instance and set as myInstance Dim myProperty = myInstance.GetType().GetProperty(FieldObject(1))