onclick get data from database using ajax laravel
Solution 1
You don't need to find designId. You just need to do
public function calcList($id) {
$design = design::select( `design_no`, `design_name`, `weight_length`, `quantity`, `design_image`, `des2`, `des3`, `des4`, `des5`, `des6`, `des7`)->where('id',$id)->get();
return Response::json($design);
}
You should not make another query to database by first finding a single design with id then using it again to get design_no, design_name, ... etc. It seems redundant.
Solution 2
First, you'd better use GET
method with cache: false
ajax setting. In this case you don't need to pass csrf
token.
Second, you forgot to use get()
method to get data. Third, you can use where
without =
, it is by default.
use Response; // don't forget or use response()
public function calcList($id)
{
$designId=design::find($id);
$design = design::select( 'design_no', 'design_name', 'weight_length', 'quantity', 'design_image', 'des2', 'des3', 'des4', 'des5', 'des6', 'des7')->where('id', $designId)->get();
return Response::json($design);
}
You can display your results as a table as follows.
<div id="destination"></div>
...
$.ajax({
type:"POST",
url:"/calc_list/"+id,
success : function(results) {
var $table = $('<table></table>');
$('#destination').html('');
for(var i=0;i<=results.length;i++) {
$table.append('<tr><td>No</td><td>'+results[i].design_no+'</td></tr>');
$table.append('<tr><td>Name</td><td>'+results[i].design_name+'</td></tr>');
}
$('#destination').append($table);
}
});
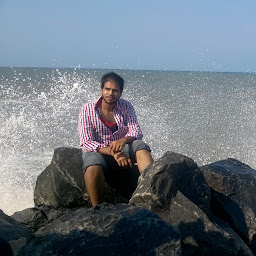
Harish Karthick
Updated on June 28, 2022Comments
-
Harish Karthick almost 2 years
What I am trying to do is to load
data-id
in drop-down menu when I click the list item, it need retrieve the data from db and append in table.So far, I loaded the data id in drop-down menu and while on-click the list I send ajax call to retrieve data.
My code (controller page):
public function calcList($id) { $designId=design::find($id); $design = design::select( `design_no`, `design_name`, `weight_length`, `quantity`, `design_image`, `des2`, `des3`, `des4`, `des5`, `des6`, `des7`)->where('id','=',$designId); // return Response::json(array('datas' => $design)); // return response()->json(['data'=>$design]); return Response::json($design); }
Ajax Request:
$(document).ready(function() { $('.designList').click(function() { $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); var id =($(this).attr("data-id")); $.ajax({ type:"POST", url:"/calc_list/"+id, success : function(results) { console.log(results); } }); }); });
On the console log, the results print like {..}
How to built the json to table?