OOP - Sessions and PHP
Solution 1
Session is essentially a variable you can store whatever you want in them, A Number, Character, Characters(String), Structs ..... Objects.
It is also a variable that remains constant through the pages. Its most commanly used to keep user logged information
How i use Session objects is as follows:
Lets say i have a users table in my DB and i need to display the firstname and lastname mostly all of my pages.
What i can do are two things... The Old way:
$result = mysql_fetch_array(mysql_query("SELECT * FROM users WHERE userId=1"));
$_SESSION['userId'] = $result['userId'];
$_SESSION['firstname'] = $result['firstname'];
$_SESSION['lastname'] = $result['lastname'];
Now you can do this which is fine but it would be alot better if you had lets say the following code:
class user{
_construct($userId){
$qry_str = mysql_query("SELECT * FROM users WHERE Id=$userId");
$result = mysql_fetch_array($qry_str);
$this->userId = $userId;
$this->firstname = $result['firstname'];
$this->lastname = $result['lastname'];
}
public $userId, $firstname, $lastname;
}
And to initialize
$_SESSION['user'] = new user(1);
Or say Multiple users:
$_SESSION['user'][1] = new user(1);
$_SESSION['user'][2] = new user(2);
$_SESSION['user'][3] = new user(3);
And then in your code all you need to do is: assign the user by userId at login and then use the class that is stored to display all your information.
There are alot more powerfull things you can do with classes. But above shows you a simple and effective solution.
Try this: Add if statments and die statments in your construct for the user class... that when constructing the user you can error check
Hope this Helps
Solution 2
HTTP is stateless. If you want data to persist between different page fetches, you need some other mechanism. Cookies are one way, but since they're stored on the client, they're not trustworthy on the server. $_SESSION
is one approach to persistent, trustworthy storage. The most common usage is for login systems. Note that session IDs come from the client, so they're not trustworthy, but you can be fairly certain the data stored within the session itself isn't spoofed. Another option would be to use a database, but then you'd have to add code to serialize/unserialize or otherwise convert data stored in the database to and from PHP objects. One advantage databases have over sessions is that data can't easily be shared between sessions.
As for storing objects as opposed to other types, it's just a matter of what your particular application uses, but it's not a necessity. Taking the login example, if you're storing authenticated user information (such as user ID and client IP address) in an object, you could store that object within $_SESSION
.
For another example, suppose you're writing a messaging application (e.g. forum, e-mail) that uses objects to model messages. Messages can have attachments, but the attachment form is a separate page, so you store the message object in $_SESSION
. Not the most usable design, but a common one, though less so with the advent of AJAX.
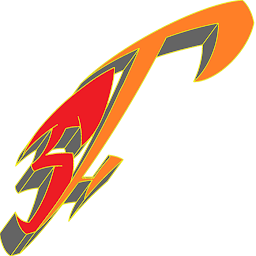
fer sid
If this Infrastructure works, it was build by me - if not .. eh someone else! When the sun shines: Working as one man DevOps Crew in Hamburg (StartUp). In love with Docker, Git, PHP (got a certification decades ago). I prefer Cloud, don't like Baremetal stuff. Deep into AWS and Azure.
Updated on June 04, 2022Comments
-
fer sid almost 2 years
iam a litte bit confused, i've started to learn php 5 the OO Way last year. First Projects are done with the Zend Framework.
But some of my friends are talking about storing Objects in the $_SESSION Superglobal Array. But iam not able to find an good example, why or when this is nesecarry ?
It would be great , if someone people can give me a hint , why to store objects in sessions.