Open new web page in new tab in WebBrowser control
Solution 1
private void Browser_ProgressChanged(object sender, WebBrowserProgressChangedEventArgs e)
{
var webBrowser = (WebBrowser)sender;
if (webBrowser.Document != null)
{
foreach (HtmlElement tag in webBrowser.Document.All)
{
if (tag.Id == null)
{
tag.Id = String.Empty;
switch (tag.TagName.ToUpper())
{
case "A":
{
tag.MouseUp += new HtmlElementEventHandler(link_MouseUp);
break;
}
}
}
}
}
}
private void link_MouseUp(object sender, HtmlElementEventArgs e)
{
var link = (HtmlElement)sender;
mshtml.HTMLAnchorElementClass a = (mshtml.HTMLAnchorElementClass)link.DomElement;
switch (e.MouseButtonsPressed)
{
case MouseButtons.Left:
{
if ((a.target != null && a.target.ToLower() == "_blank") || e.ShiftKeyPressed || e.MouseButtonsPressed == MouseButtons.Middle)
{
AddTab(a.href);
}
else
{
CurrentBrowser.TryNavigate(a.href);
}
break;
}
case MouseButtons.Right:
{
CurrentBrowser.ContextMenuStrip = null;
var contextTag = new ContextTag();
contextTag.Element = a;
contextHtmlLink.Tag = contextTag;
contextHtmlLink.Show(Cursor.Position);
break;
}
}
}
See more at dotBrowser.sf.net project
Solution 2
This seems to be a common problem with the browser control. It's not really a fully-functional browser and things like tabbed browsing and pop-ups are a pain with it.
A lot of things I find online simply say "you can't do that with this control." Kind of a cop-out, really. But I have in the past stumbled across this project which extends the control to add additional functionality. It's kind of old, and I haven't actually used it. But it claims to have additional support for targeted links by means of "tabs" or MDI controls. Might be worth a try.
Solution 3
There is two ways you can work around on this. Both methods begin with capturing the click event and then detecting whether an "a" element is clicked.
Method 1 simply gets the URL, cancel the click, and have the open a new tab. Opening a new tab may be achieved by simply instantiating a new WebBrowser control at the right place.
Method 2 simply removes the _blank
from the target
so that the page opens on the current browser rather than spawning another browser window.
private void Go(string url)
{
webBrowser1.Navigate(url);
webBrowser1.DocumentCompleted += new WebBrowserDocumentCompletedEventHandler(webBrowser1_DocumentCompleted);
}
void webBrowser1_DocumentCompleted(object sender, WebBrowserDocumentCompletedEventArgs e)
{
webBrowser1.Document.Click += new HtmlElementEventHandler(Document_Click);
}
void Document_Click(object sender, HtmlElementEventArgs e)
{
HtmlElement ele = webBrowser1.Document.GetElementFromPoint(e.MousePosition);
while (ele != null)
{
if (ele.TagName.ToLower() == "a")
{
// METHOD-1
// Use the url to open a new tab
string url = ele.GetAttribute("href");
// TODO: open the new tab
e.ReturnValue = false;
// METHOD-2
// Use this to make it navigate to the new URL on the current browser/tab
ele.SetAttribute("target", "_self");
}
ele = ele.Parent;
}
}
However, do note that these methods don't prevent browser windows from being opened outside your application via JavaScript.
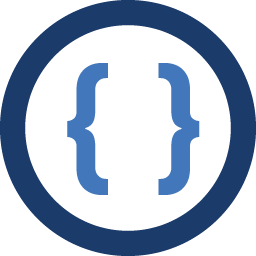
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm using
WebBrowser
control in my c# application, and I want to open web pages in it.
It's completely done.My problem:
Any link in the web page that its target is
_blank
will open in new IE Window.I'd like to open such link in new tab in my application.
How to do it?
Thanks!