(OpenCV) Getting a 'unresolved external symbol' error
I ran into a similar problem with OpenCV. You're likely missing one or more .lib files to the Linker.
You can add them by:
- Right-Clicking on your project in Visual Studio.
- Selecting "Properties"
- Take note of: "Linker > General > Additional Library Directories"
- Go to "Linker > Input > Additional Dependencies"
I specified OpenCV lib directory to VS under "Additional Library Directories". I browsed to this location and added my missing .lib files in "Additional Dependencies".
For example (I'm using 2.4.9 debug, so I added):
opencv_calib3d249d.lib
opencv_contrib249d.lib
opencv_core249d.lib
opencv_features2d249d.lib
opencv_flann249d.lib
opencv_gpu249d.lib
opencv_highgui249d.lib
opencv_imgproc249d.lib
opencv_legacy249d.lib
opencv_ml249d.lib
opencv_nonfree249d.lib
opencv_objdetect249d.lib
opencv_ocl249d.lib
opencv_photo249d.lib
opencv_stitching249d.lib
opencv_superres249d.lib
opencv_ts249d.lib
opencv_video249d.lib
opencv_videostab249d.lib
If you're using release, make sure to use only the release libs!
You might have to do something similar depending on how you setup your project.
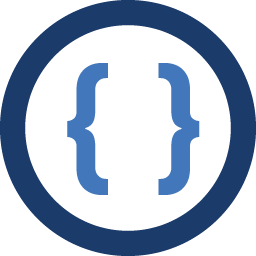
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
I'm using this tutorial (from http://hxr99.blogspot.com/2011/12/opencv-examples-showing-image.html), but many errors show up:
#include <iostream> #include <opencv2\opencv.hpp> using namespace std; using namespace cv; int main( int argc, const char** argv ) { Mat image; String inputName; for( int i = 1; i < argc; i++ ) { inputName.assign( argv[i] ); } if( inputName.empty() || (isdigit(inputName.c_str()[0]) && inputName.c_str()[1] == '\0') ) { if( inputName.size() ) { image = imread( inputName, 1 ); } else { if(image.empty()) cout << "Couldn't read image" << endl; } imshow("Test",image); } }
Errors:
1>------ Build started: Project: Project1, Configuration: Debug x64 ------ 1> main.cpp 1>main.obj : error LNK2019: unresolved external symbol "void __cdecl cv::fastFree(void *)" (?fastFree@cv@@YAXPEAX@Z) referenced in function "public: __cdecl cv::Mat::~Mat(void)" (??1Mat@cv@@QEAA@XZ) 1>main.obj : error LNK2019: unresolved external symbol "public: __cdecl cv::_InputArray::_InputArray(class cv::Mat const &)" (??0_InputArray@cv@@QEAA@AEBVMat@1@@Z) referenced in function main 1>main.obj : error LNK2019: unresolved external symbol "public: void __cdecl cv::Mat::deallocate(void)" (?deallocate@Mat@cv@@QEAAXXZ) referenced in function "public: void __cdecl cv::Mat::release(void)" (?release@Mat@cv@@QEAAXXZ) 1>main.obj : error LNK2019: unresolved external symbol "public: void __cdecl cv::Mat::copySize(class cv::Mat const &)" (?copySize@Mat@cv@@QEAAXAEBV12@@Z) referenced in function "public: class cv::Mat & __cdecl cv::Mat::operator=(class cv::Mat const &)" (??4Mat@cv@@QEAAAEAV01@AEBV01@@Z) 1>main.obj : error LNK2019: unresolved external symbol "int __cdecl cv::_interlockedExchangeAdd(int *,int)" (?_interlockedExchangeAdd@cv@@YAHPEAHH@Z) referenced in function "public: class cv::Mat & __cdecl cv::Mat::operator=(class cv::Mat const &)" (??4Mat@cv@@QEAAAEAV01@AEBV01@@Z) 1>main.obj : error LNK2019: unresolved external symbol "void __cdecl cv::imshow(class std::basic_string<char,struct std::char_traits<char>,class std::allocator<char> > const &,class cv::_InputArray const &)" (?imshow@cv@@YAXAEBV?$basic_string@DU?$char_traits@D@std@@V?$allocator@D@2@@std@@AEBV_InputArray@1@@Z) referenced in function main 1>main.obj : error LNK2019: unresolved external symbol "class cv::Mat __cdecl cv::imread(class std::basic_string<char,struct std::char_traits<char>,class std::allocator<char> > const &,int)" (?imread@cv@@YA?AVMat@1@AEBV?$basic_string@DU?$char_traits@D@std@@V?$allocator@D@2@@std@@H@Z) referenced in function main 1>c:\users\gerard\documents\visual studio 2012\Projects\Project1\x64\Debug\Project1.exe : fatal error LNK1120: 7 unresolved externals ========== Build: 0 succeeded, 1 failed, 0 up-to-date, 0 skipped ==========
I don't know what's happening. Any help is very very appreciated. Btw, I'm using Visual Studio 2012, and I (hope to) have the correct PATH and libraries.