opencv saving image captured from webcam
The problem is that you are mixing your pointer syntax. You are creating a new IplImage
with IplImage* img= cvCreateImage(size, IPL_DEPTH_16S, 1);
but on the following line, you lose this structure as you overwrite the pointer img
with frame
.
The code causing your sigabrt is where you're sending a pointer to a pointer in
cvSaveImage("matteo.jpg",&img);
. You should not do &img
as img
already is a pointer. The following is correct:
cvSaveImage("matteo.jpg",img);
There is actually no reason for you to create a new IplImage
unless you want to do some preprocessing before saving it to file.
I modified your if
-clause to the following which works fine on my computer:
if ( cvWaitKey(10) < 0 ) {
cvSaveImage("matteo.jpg",frame);
}
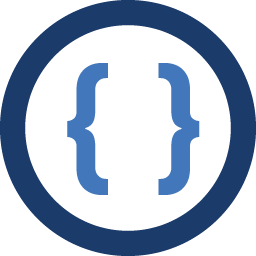
Admin
Updated on January 22, 2020Comments
-
Admin over 4 years
I receive an error "SIGABRT ERROR" when the code is trying to save the image on the HD.
I'm working with a MacBook Pro Mountain Lion on last XCODE and the libraries are well reconfigured.
Someone has a solution or some ideas?
#include "cv.h" #include "highgui.h" #include <stdio.h> using namespace cv; // A Simple Camera Capture Framework int main() { CvCapture* capture = cvCaptureFromCAM( CV_CAP_ANY ); if ( !capture ) { fprintf( stderr, "ERROR: capture is NULL \n" ); getchar(); return -1; } // Create a window in which the captured images will be presented cvNamedWindow( "mywindow", CV_WINDOW_AUTOSIZE ); // Show the image captured from the camera in the window and repeat while ( 1 ) { // Get one frame IplImage* frame = cvQueryFrame( capture ); if ( !frame ) { fprintf( stderr, "ERROR: frame is null...\n" ); getchar(); break; } cvShowImage( "mywindow", frame ); // Do not release the frame! if ( (cvWaitKey(10) & 255) == 's' ) { CvSize size = cvGetSize(frame); IplImage* img= cvCreateImage(size, IPL_DEPTH_16S, 1); img = frame; cvSaveImage("matteo.jpg",&img); } if ( (cvWaitKey(10) & 255) == 27 ) break; } // Release the capture device housekeeping cvReleaseCapture( &capture ); cvDestroyWindow( "mywindow" ); return 0; }