Opening video with openCV +python
19,063
Solution 1
Try using cv.CaptureFromFile()
instead.
Copy this code if you must: Watch Video in Python with OpenCV.
Solution 2
you can use the new interface of OpenCV (cv2), the object oriented one, which is binded from c++. I find it easier and more readable.
note: if your open a picture with this, the fps doesn't mean anything, so the picture stays still.
import cv2
import sys
try:
vidFile = cv2.VideoCapture(sys.argv[1])
except:
print "problem opening input stream"
sys.exit(1)
if not vidFile.isOpened():
print "capture stream not open"
sys.exit(1)
nFrames = int(vidFile.get(cv2.cv.CV_CAP_PROP_FRAME_COUNT)) # one good way of namespacing legacy openCV: cv2.cv.*
print "frame number: %s" %nFrames
fps = vidFile.get(cv2.cv.CV_CAP_PROP_FPS)
print "FPS value: %s" %fps
ret, frame = vidFile.read() # read first frame, and the return code of the function.
while ret: # note that we don't have to use frame number here, we could read from a live written file.
print "yes"
cv2.imshow("frameWindow", frame)
cv2.waitKey(int(1/fps*1000)) # time to wait between frames, in mSec
ret, frame = vidFile.read() # read next frame, get next return code
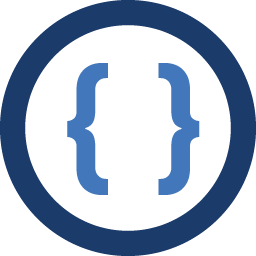
Author by
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I using python 2.7 and openCV 2.3.1 (win 7). I trying open video file:
stream = cv.VideoCapture("test1.avi") if stream.isOpened() == False: print "Cannot open input video!" exit()
But I have warning:
warning: Error opening file (../../modules/highgui/src/cap_ffmpeg_impl_v2.hpp:394)
If use video camera (
stream = cv.VideoCapture(0)
), this code works. Any ideas as to what I'm doing wrong? Thanks a lot to all!