Operator << for QString
Solution 1
If the <<
operator is included in the Qt library every client of the library will have to use the exact same implementation. But due to the nature of QString it is far from obvious this is what these clients want. Some people writing software interacting with legacy file in western europe may want to use Latin1() characters, US people may go with Ascii() and more modern software may want to use Utf8().
Having a single implementation in the library would restrict unacceptably what can be done with the whole library.
Solution 2
It's not necessary to implement such thing, as long as there exists a convenient solution like this one, involving QTextStream
QString s;
QTextStream out(&s);
out << "Text 1";
out << "Text 2";
out << "And so on....";
QTextStream is quite powerfull...
Solution 3
The accepted answer points out some valid reasons for why there is no operator<<
function for QString
.
One can easily overcome those reasons by providing some convenience functions and maintaining some state in an application specific namespace
.
#include <iostream>
#include <QString>
namespace MyApp
{
typedef char const* (*QStringInsertFunction)(QString const& s);
char const* use_toAscii(QString const& s)
{
return s.toAscii().constData();
}
char const* use_toUtf8(QString const& s)
{
return s.toUtf8().constData();
}
char const* use_toLatin1(QString const& s)
{
return s.toLatin1().constData();
}
// Default function to use to insert a QString.
QStringInsertFunction insertFunction = use_toAscii;
std::ostream& operator<<(std::ostream& out, QStringInsertFunction fun)
{
insertFunction = fun;
return out;
}
std::ostream& operator<<(std::ostream& out, QString const& s)
{
return out << insertFunction(s);
}
};
int main()
{
using namespace MyApp;
QString testQ("test-string");
std::cout << use_toAscii << testQ << std::endl;
std::cout << use_toUtf8 << testQ << std::endl;
std::cout << use_toLatin1 << testQ << std::endl;
return 0;
}
Output:
test-string
test-string
test-string
Solution 4
I don't think there is any particular reason for excluding (nor including) this in the Qt library
. Only problem that could possibly appear here is a possibility that std::ostream
object could modify the contents of the parameter passed to std::ostream::operator<<
function.
However, in the reference it is clearly stated that this function will modify the parameter if string buffer is passed - there is nothing about the other types, so I guess (and the common-sense is telling me) that operator<< will not modify char*
parameter. Also, on this page there is nothing about modifying the passed object.
Last thing: instead of using QString::toAscii().constData()
, you could use QString::toStdString()
or qPrintable(const QString&)
macro.
Related videos on Youtube
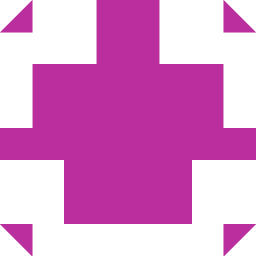
Ilya Kobelevskiy
Updated on September 14, 2022Comments
-
Ilya Kobelevskiy 9 months
It makes sense to implement << for QString like:
std::ostream& operator <<(std::ostream &stream,const QString &str) { stream << str.toAscii().constData(); //or: stream << str.toStdString(); //?? return stream; }
instead of writing
stream << str.toAscii().constData();
every time in the code.
However, since it is not in standard Qt library, I'm assuming there is any particular reason not to do so. What are the risks/inconvenience of overloading << as specified above?
-
fjardonI don't see why it makes sense to use str.toAscii() instead of toLatin1() or toUtf8() or toLocal8Bit() ?
-
Yakk - Adam NevraumontThey do very different things outside of 7-bit clean strings.
-
Angew is no longer proud of SOI believe the reason it's not in Qt is that Qt is pretty much trying to replace the entire standard library with itself. Not that I like this philosophy of theirs, but they seem keen on it.
-