Opposite of Java .equals() method?
Solution 1
The exclamation point !
represents negation or compliment. while(!"jack".equals(name)){ }
Solution 2
The boolean not ! operator is the cleanest way. Of course you could also say
if ("jack".equals(name) == false) { ... }
or you could say
if ("jack".equals(name) != true) { ... }
Beware if calling .equals()
on an object that could be null
or you'll get a NullPointerException
. In that case, something like...
if !((myVar == yourVar) || ((yourVar != null) && yourVar.equals(myVar))) { ... }
... would guard against an NullPointerException
and be true if they're no equal, including them both not being null
. Quite a brain-twister huh? I think that logic is sound, if ugly. That's why I write a StringUtil
class containing this logic in many projects!
That's why it's a better convention to invoke the .equals()
method on the string literal rather than the String you're testing.
Solution 3
Put the "!" in from of jack. That will solve if I understand the question correctly.
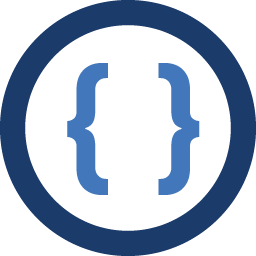
Admin
Updated on July 21, 2022Comments
-
Admin almost 2 years
I'm trying to write a little block of code in Java with a do-while loop that asks the user's name. If it isn't the name the code is looking for, then it just repeats itself. For example,
Scanner scan = new Scanner(); do { System.out.println("whats your name?"); String name = scan.nextLine(); } while ("jack".equals(name)); ////// <<<<
It's where I marked with
<<<<
that I don't know what to do. I need something like!=
, but that does not work with strings.So what can I put here?
-
Mike Nitchie almost 11 yearsMy mistake. Edited for accuracy.
-
FThompson almost 11 years@acdcjunior
jack
appeared to be a variable in the original, poor formatting of the question. -
acdcjunior almost 11 years@Vulcan I was talking about the original one, actually. It was
"jack
then (thus the seems). Right now it clearly is aString
. -
acdcjunior almost 11 years+1 almost too many info, but correct! Tip: format you code using CTRL+K, makes it much easier to read.