Optional get value if present
Solution 1
If you have a default value for your Desktop
, you could try with Optional.orElse
:
Desktop defaultDesktop = ...;
Desktop desktop = Optional.ofNullable(status)
.map(Status::getDesktop)
.orElse(defaultDesktop);
However, you don't have to necessarily work inside a lambda expression with Optional.ifPresent
. You could perfectly use a method that receives a Desktop
instance, which would act as the Consumer
argument of Optional.ifPresent
:
Desktop desktop = Optional.ofNullable(status)
.map(Status::getDesktop)
.ifPresent(this::workWithDesktop);
Then:
void workWithDesktop(Desktop desktop) {
// do whatever you need to do with your desktop
}
If you need additional arguments (apart from the desktop itself), you could use a lambda expression that invokes the method instead:
String arg1 = "hello";
int arg2 = 10;
Desktop desktop = Optional.ofNullable(status)
.map(Status::getDesktop)
.ifPresent(desktop -> this.workWithDesktop(desktop, arg1, arg2));
And then:
void workWithDesktop(Desktop desktop, String arg1, int arg2) {
// do whatever you need to do with your desktop, arg1 and arg2
}
Solution 2
I was told here just last week that it's a code smell to even use Optional like this at all, so
Desktop desktop = (status != null)? status.getDesktop() : null;
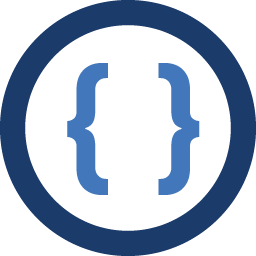
Admin
Updated on June 22, 2022Comments
-
Admin almost 2 years
As an example I have an optional like this:
Optional<Desktop> opt = Optional.ofNullable(status).map(Status::getDesktop);
I want to have the desktop and work with it outside of lambda expressions. I do it like this:
if (opt.isPresent()){ Desktop desktop = opt.get(); ... }
Is there a better solution to get the desktop; something like this ?
Desktop desktop = Optional.ofNullable(status).map(Status::getDesktop).ifPresent(get());
EDIT: OrElse was the method I was looking for :)