Order of controls in a form's Control property in C#
Solution 1
look at the order in which they are added to the form in the yourForm.designer.cs
Solution 2
I know this is quite an old question, but...
You might want to use SetChildIndex
. e.g. this.Controls.SetChildIndex(button1, 0);
Solution 3
if you look at the code generated by the designer Form1.designer.cs it will look something like this:
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(658, 160);
this.Controls.Add(this.flowLayoutPanel7);
this.Controls.Add(this.flowLayoutPanel6);
this.Controls.Add(this.flowLayoutPanel5);
this.Controls.Add(this.flowLayoutPanel4);
this.Controls.Add(this.flowLayoutPanel3);
this.Controls.Add(this.flowLayoutPanel2);
this.Controls.Add(this.flowLayoutPanel1);
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
note how it was built up you added panel 1 first then 2 etc. but as the code runs through it will add 7 first then 6.
this code will be in the InitializeComponent() function generated by the designer.
Why do you need them to run in a certain order?
I wouldn't rely on the designer to keep the order you want.. i would sort the controls my self:
var flowpanelinOrder = from n in this.Controls.Cast<Control>()
where n is FlowLayoutPanel
orderby int.Parse(n.Tag.ToString())
select n;
/* non linq
List<Control> flowpanelinOrder = new List<Control>();
foreach (Control c in this.Controls)
{
if (c is FlowLayoutPanel) flowpanelinOrder.Add(c);
}
flowpanelinOrder.Sort();
* */
foreach (FlowLayoutPanel aDaysControl in flowpanelinOrder)
{
MessageBox.Show(aDaysControl.Tag.ToString());
}
Solution 4
What if in future some other designer removed the controls, added back etc? Checking the designer always is a mess. What would be better is to sort the controls in the container control before you enumerate. I use this extension method (if you have Linq):
public static List<Control> ToControlsSorted(this Control panel)
{
var controls = panel.Controls.OfType<Control>().ToList();
controls.Sort((c1, c2) => c1.TabIndex.CompareTo(c2.TabIndex));
return controls;
}
And you can:
foreach (FlowLayoutPanel aDaysControl in this.ToControlsSorted())
{
MessageBox.Show(aDaysControl.TabIndex.ToString());
}
(Above is for TabIndex
). Would be trivial to sort according to Tag
from that.
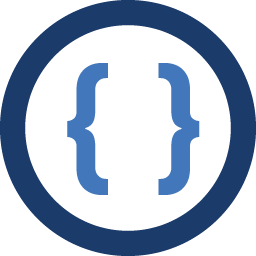
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
I am having a peculiar problem with the order in which FlowLayoutPanels are added in to the form's controls property. This is what I tried,
I added 7 FlowLayoutPanels in to a C# window application from left to right in vertical strips. Then I tagged the flow layouts as 1, 2, 3, ... 7 again from left to right. Now in the load handler of the form, I wrote the following snippet,
foreach (FlowLayoutPanel aDaysControl in this.Controls) { MessageBox.Show(aDaysControl.Tag.ToString()); }
I expected messages to appear in the order of 1, 2, ... 7. But I got it in the reverse order (7, 6, ...1). Could some one help me out with the mistake I did ??
Reason behind preserving the order,
I am trying to make a calendar control with each row representing a day. If a month starts from Wednesday, then I need to add a empty label to the first(Monday) and the second(Tuesday) row. So the order matters a bit
-
Admin over 15 yearsBut the order does_ matter in my case !!
-
Admin over 15 yearsYeah. Thats that problem. They were added in the opposite way. Don't know why. Thanks
-
Admin over 15 yearsI am trying to make a calendar control with each row representing a day. If a month starts from Wednesday, then I need to add a empty label to the first(Monday) and the second(Tuesday) row. So the order matters a bit.
-
Admin over 15 yearsHmm. I was bit lazy. Learn the lesson now.