Order of operations for pre-increment and post-increment in a function argument?
Solution 1
Well, there are two things to consider with your example code:
- The order of evaluation of function arguments is unspecified, so whether
++a
ora++
is evaluated first is implementation-dependent. - Modifying the value of
a
more than once without a sequence point in between the modifications results in undefined behavior. So, the results of your code are undefined.
If we simplify your code and remove the unspecified and undefined behavior, then we can answer the question:
void xyz(int x) { }
int a = 1;
xyz(a++); // 1 is passed to xyz, then a is incremented to be 2
int a = 1;
xyz(++a); // a is incremented to be 2, then that 2 is passed to xyz
Solution 2
Quoting Kernighan & Ritchie, Chapter 2.12:
The order in which function arguments are evaluated is not specified, so the statement
printf("%d %d\n", ++n, power(2, n)); /* WRONG */
can produce different results with different compilers, depending on whether n is incremented before power is called. The solution, of course, is to write
++n; printf("%d %d\n", n, power(2, n));
Function calls, nested assignment statements, and increment and decrement operators cause ``side effects'' - some variable is changed as a by-product of the evaluation of an expression. In any expression involving side effects, there can be subtle dependencies on the order in which variables taking part in the expression are updated. One unhappy situation is typified by the statement
a[i] = i++;
The question is whether the subscript is the old value of i or the new. Compilers can interpret this in different ways, and generate different answers depending on their interpretation. The standard intentionally leaves most such matters unspecified. When side effects (assignment to variables) take place within an expression is left to the discretion of the compiler, since the best order depends strongly on machine architecture. (The standard does specify that all side effects on arguments take effect before a function is called, but that would not help in the call to printf above.) The moral is that writing code that depends on order of evaluation is a bad programming practice in any language. Naturally, it is necessary to know what things to avoid, but if you don't know how they are done on various machines, you won't be tempted to take advantage of a particular implementation.
Solution 3
Unary Operator evaluation sequence for a function:
#include <stdio.h>
void xyz(int x, int y) {
printf("x:%d y:%d ", x, y);
}
main() {
int a;
a=1; xyz(++a, a); printf("a:%d\n", a);
a=1; xyz(a, a++); printf("a:%d\n", a);
a=1; xyz(++a, a++); printf("a:%d\n", a);
}
will output
x:2 y:2 a:2
x:2 y:1 a:2
x:3 y:1 a:3
On my system. This indicates that the second parameter of the function is being evaluated first. You should not rely on order of evaluation of function parameters. It is not defined, so it will be different on different systems.
Good job on finding a nifty example of this behavior, though.
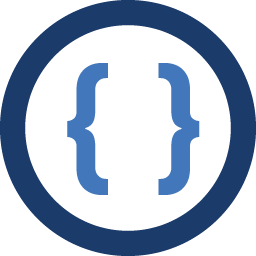
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I have some C code:
main() { int a=1; void xyz(int,int); xyz(++a,a++); //which Unary Operator is executed first, ++a or a++? printf("%d",a); } void xyz(int x,int y) { printf("\n%d %d",x,y); }
The function
xyz
has two parameters passed in,++a
anda++
. Can someone explain the sequence of operations to explain the result?The above code prints "3 13" or "2 23" depending on which compiler is used.
-
John Bode almost 14 yearsIs it true in the second case that
a
is updated before the function is called? I know the result of the expression++a
is 2, which is what gets passed toxyz
, but my understanding was that the side effect may not necessarily be applied before the function is called. -
James McNellis almost 14 years@John: Yes: there is a sequence point after the evaluation of all of the arguments to a function but before the function is called.