OrderBy Date values, which are just strings in Angular JS
Because orderBy
filter, you can use that.
Your ng-repear would probably look similar to this:
ng-repeat="item in items | orderBy: orderByDate"
and then on your controller you would define the orderByDate
function:
$scope.orderByDate = function(item) {
var parts = item.dateString.split('-');
var date = new Date(parseInt(parts[2],
parseInt(parts[1]),
parseInt(parts[0]));
return date;
};
Implementation of the function is up to you. I choose to create Date
from the string. The object you return from orderByDate
function is then used to be order with using <, =, > operators.
EDIT: solution for :reverse
Since :reverse
can't be used with a function passed as parameter, you can implement your custom function to return reversed value itself. In such case using Date
is not possible, I would construct a number then and returned it with minus:
$scope.orderByDate = function(item) {
var parts = item.dateString.split('-');
var number = parseInt(parts[2] + parts[1] + parts[0]);
return -number;
};
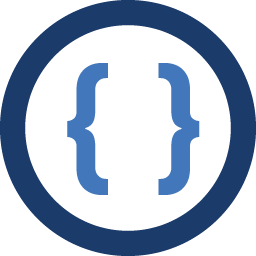
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
I'm trying to order some data by date, although the dates are just strings, in the format dd-mm-yyyy.
I made a filter which converted the plain string of numbers (which where in the US date format, where I wanted the UK date format) e.g 01272012 to 27-01-2014, but when I try to order them it still only sees them as numeric strings, so 01-01-1990 would come before 02-01-2014.
Any suggestions of how to do this in a filter?
Thanks!
Update
I figured out the dates would automatically get orderedif the date format was yyyy-mm-dd. I then used used
orderBy:['date']
to order the data, only using my original filter when displaying the data.I ended up having to reverse my data, showing the most recent dates. To achieve this I added a
-
to my orderBy statment:orderBy:['-date']
. -
Admin over 9 yearsAwesome, I think I've got it working. My set up is slightly different - I'm passing a paramter into the orderByDate function suggested by yourself, how would one reverse it? I'm getting an error when using ':reverse' after the method.
-
David Bohunek over 9 yearsI see,
:reverse
doesn't work when a function is passed. The I suggest construction anumber
rather thanDate
and returning it with with minus. Will update my answer -
David Bohunek over 9 yearsUpdated with solution for
:reverse
at the bottom. -
Admin over 9 yearsThanks for your detailed and speedy replies. I figured out if the date is ordered as year-month-day, then the number will automatically be sorted in the correct order, so the date 19990812 would come before 20000912. I've just applied my original filter on these to display them how I want but all I had to do was:
orderBy:['-date']
. Thanks again.