Outputting a Unicode character in C#
Solution 1
Why are you converting it to unicode, this will not do anything.. lose the conversion and do the following:
string a ="\u2649" ;
Console.write(a) ;
Solution 2
You need to have a font which displays that glyph. If you do, then:
Console.WriteLine(myString);
is all you need.
EDIT: Note, the only font I could find which has this glyph is "MS Reference Sans Serif".
Solution 3
The length of the Unicode character, in bytes, is 2 and you are writing the Length
to the Console.
Console.WriteLine(unicode.Length);
If you want to display the actual character, then you want:
Console.WriteLine(myString);
You must be using a font that has that Unicode range for it to display properly.
UPDATE:
Using default console font the above Console.WriteLine(myString)
will output a ?
character as there is no \u2649
. As far I have so far googled, there is no easy way to make the console display Unicode characters that are not already part of the system code pages or the font you choose for the console.
It may be possible to change the font used by the console: Changing Console Fonts
Solution 4
You are outputting the length of the character, in bytes. The Console doesn't support unicode output, however, so it will come out as an '?' character.
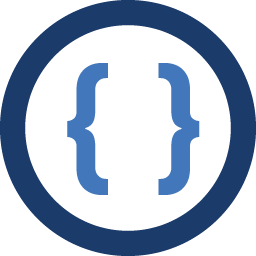
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm new to programming and self taught. I'm trying to output the astrological symbol for Taurus, which is supposed to be U+2649 in Unicode. Here is the code I'm using...
string myString = "\u2649"; byte[] unicode = System.Text.Encoding.Unicode.GetBytes(myString); Console.WriteLine(unicode.Length);
The result I'm getting is the number 2 instead of the symbol or font. I'm sure I'm doing something wrong.
-
Admin almost 14 yearsDoes the default console font contain all unicode characters?
-
codekaizen almost 14 yearsNo. I looked at the most developed fonts in Character Map and found that MS Reference Sans Serif has it. None of the other usual suspects - Arial, Verdana, Tahoma, Calibri - have it. MS Reference Sans Serif is not a fixed-size glyph, though, so it will look odd in Console.
-
Rup almost 14 yearsArial Unicode does, although you probably have to tick some extra boxes to install it (or install Office?), as do MS Mincho/PMincho and MS Gothic/PGothic. Now I generally do everything on the console but if you want complex characters like this you probably ought to build a GUI app instead.
-
Dennis almost 14 years@Hans: sorry, no I did not. I should have put a disclaimer. I will try it now and post an update to my answer. Thanks Hans.
-
Admin almost 14 yearsThanks to all those who have responded. Ed
-
Admin almost 14 yearsThanks Dennis. That was very helpful. While I don't understand all of the coding for changing the Console Fonts, it is quite interesting.
-
Dennis almost 14 years@Ed: Why does it have to a console output? It would easier to create a simple form with a text box as you would be able to set the font.
-
Admin almost 14 yearsHi Dennis, Hope you had a good holiday. I'm just playing around trying to learn C#. I'm really new to this and thought it would be fun to write a simple program to output astrological signs. I've seen them on the internet and wondered how they output them. I guess they are using HTML? If I do find a character set that has the glyphs, how do I include it in my program? Do I put the file in the reference folder on Visual C# 2008 Express and use a using statement? I really don't want to output a jpeg or anything like that. Thanks Dennis, -Ed-