Overloading operator<< - must be a binary operator
11,762
The operator should be declared either as a friend function of the class
friend std::ostream& operator<<(std::ostream& os, const domino & dom);
or you should remove the operator declaration from the class definition.
Otherwise the compiler considers the operator as a member function of the class.
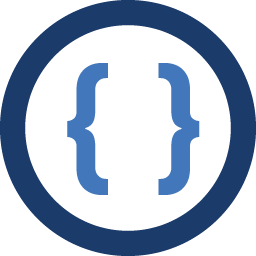
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
What's the error here? I reviewed prior Q&A, but all those coders appear to have made other errors when overloading <<. When I try it, QT Creator gives this error:
overloaded 'operator<<' must be a binary operator (has 3 parameters)
, referring to the line in the.h
file.Edited code below...
domino.h:
#include <string> #include <iostream> class domino { public: domino(); domino(int leftDots, int rightDots); std::string toString() const; std::ostream& operator<<(std::ostream& os, const domino & dom); private: int leftDots; /* Dots on left side */ int rightDots; /* Dots on right side */ }; #endif
domino.cpp:
#include "domino.h" #include <string> domino::domino() { this->leftDots = 0; this->rightDots = 0; } domino::domino(int leftNum, int rightNum) { this->leftDots = leftNum; this->rightDots = rightNum; } std::string domino::toString() const { return "[ " + std::to_string(leftDots) + "|" + std::to_string(rightDots) + " ]"; } std::ostream& operator<<(std::ostream& os, const domino & dom) { return os << dom.toString(); }
main.cpp:
#include "domino.h" #include "domino.cpp" #include <iostream> int main() { domino dom; std::cout << dom << std::endl; for(int i = 0; i < 7; i++) { for(int j = i; j < 7; j++) { domino newDom(i,j); std::cout << newDom << std::endl; } } return 0; }