Override delete operator
12,127
Solution 1
As the error message indicates, you can't delete
a void*
. Try this:
// See http://www.informit.com/guides/content.aspx?g=cplusplus&seqNum=40
#include <new> // for size_t
class Complex
{
public:
Complex() {}
~Complex() {}
static void* operator new (size_t size) {
return new char[size];
}
static void operator delete (void *p) {
return delete[] static_cast<char*>(p);
}
};
int main () {
Complex *p = new Complex;
delete p;
}
Solution 2
Your declarations are correct. The problem is in the code that implements your operator delete
: it uses the keyword delete
instead of calling the global operator delete
. Write it like this:
void Complex::operator delete(void *ptr) {
::operator delete(ptr);
}
That's assuming that your operator new
used the global operator new
.
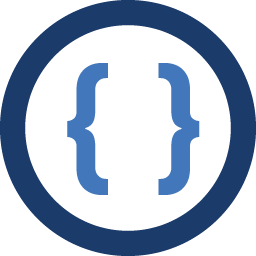
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I want to override delete operator in my class. Here's what I am trying to do,but not succeeding.
class Complex{ void *operator new(size_t s); void operator delete(void *ptr); }; void Complex::operator delete(void *ptr){ delete ptr; }
I get the error:
deleting void* is undefined
-
Admin about 11 yearsSo, may i know what is happening in those two lines?
-
GManNickG about 11 yearsUse
static_cast
here, notreinterpret_cast
. -
Admin about 11 yearsSo, in the new method does it suffice to write : return (::operator new(s));
-
Pete Becker about 11 years@AntonioKumar - yes, the static member function can be written to call the global
operator new
like that. Which, of course, raises the question of why it's there, since it just does what the compiler would do in its absence. -
GManNickG about 11 years@EmilioGaravaglia: Use a
static_cast
to undo implicit conversions (char*
implicitly converts tovoid*
),reinterpret_cast
is too strong. You aren't reinterpreting anything, just rightfully undoing an implicit cast.