Parse JSON file using GSON
Solution 1
Imo, the best way to parse your JSON response with GSON would be creating classes that "match" your response and then use Gson.fromJson()
method.
For example:
class Response {
Map<String, App> descriptor;
// standard getters & setters...
}
class App {
String name;
int age;
String[] messages;
// standard getters & setters...
}
Then just use:
Gson gson = new Gson();
Response response = gson.fromJson(yourJson, Response.class);
Where yourJson
can be a String
, any Reader
, a JsonReader
or a JsonElement
.
Finally, if you want to access any particular field, you just have to do:
String name = response.getDescriptor().get("app3").getName();
You can always parse the JSON manually as suggested in other answers, but personally I think this approach is clearer, more maintainable in long term and it fits better with the whole idea of JSON.
Solution 2
I'm using gson 2.2.3
public class Main {
/**
* @param args
* @throws IOException
*/
public static void main(String[] args) throws IOException {
JsonReader jsonReader = new JsonReader(new FileReader("jsonFile.json"));
jsonReader.beginObject();
while (jsonReader.hasNext()) {
String name = jsonReader.nextName();
if (name.equals("descriptor")) {
readApp(jsonReader);
}
}
jsonReader.endObject();
jsonReader.close();
}
public static void readApp(JsonReader jsonReader) throws IOException{
jsonReader.beginObject();
while (jsonReader.hasNext()) {
String name = jsonReader.nextName();
System.out.println(name);
if (name.contains("app")){
jsonReader.beginObject();
while (jsonReader.hasNext()) {
String n = jsonReader.nextName();
if (n.equals("name")){
System.out.println(jsonReader.nextString());
}
if (n.equals("age")){
System.out.println(jsonReader.nextInt());
}
if (n.equals("messages")){
jsonReader.beginArray();
while (jsonReader.hasNext()) {
System.out.println(jsonReader.nextString());
}
jsonReader.endArray();
}
}
jsonReader.endObject();
}
}
jsonReader.endObject();
}
}
Solution 3
One thing that to be remembered while solving such problems is that in JSON file, a {
indicates a JSONObject
and a [
indicates JSONArray
. If one could manage them properly, it would be very easy to accomplish the task of parsing the JSON file. The above code was really very helpful for me and I hope this content adds some meaning to the above code.
The Gson JsonReader documentation explains how to handle parsing of JsonObjects
and JsonArrays
:
- Within array handling methods, first call beginArray() to consume the array's opening bracket. Then create a while loop that accumulates values, terminating when hasNext() is false. Finally, read the array's closing bracket by calling endArray().
- Within object handling methods, first call beginObject() to consume the object's opening brace. Then create a while loop that assigns values to local variables based on their name. This loop should terminate when hasNext() is false. Finally, read the object's closing brace by calling endObject().
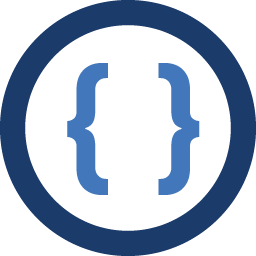
Admin
Updated on July 12, 2020Comments
-
Admin almost 4 years
I want to parse this JSON file in JAVA using GSON :
{ "descriptor" : { "app1" : { "name" : "mehdi", "age" : 21, "messages": ["msg 1","msg 2","msg 3"] }, "app2" : { "name" : "mkyong", "age" : 29, "messages": ["msg 11","msg 22","msg 33"] }, "app3" : { "name" : "amine", "age" : 23, "messages": ["msg 111","msg 222","msg 333"] } } }
but I don't know how to acceed to the root element which is : descriptor, after that the app3 element and finally the name element.
I followed this tutorial http://www.mkyong.com/java/gson-streaming-to-read-and-write-json/, but it doesn't show the case of having a root and childs elements.
-
Admin almost 11 yearsThank you :) but why do we have to set
jsonReader.beginObject();
andjsonReader.endObject();
in each method ? (main and readApp) Thank you :) -
tucuxi almost 11 yearsI believe this captures the spirit of JSON much better than a "manual parser" approach
-
Gere almost 11 yearsI agree with miko. GSON is a very powerful library for convert JSON-Java Object and viceversa.. I have used it that way in most projects.
-
Admin almost 11 yearsThank you, but the JSON file will not contain only app1, app2, app3, it will be dynamic, so I will not be able to fix the class attributes..
-
MikO almost 11 years@Mehdi see edited code, which is even simpler and allows you to have an arbitrary number of app objects in your JSON response, just changing the tipe for a
Map
... Btw, if you'll have an arbitrary number of app objects, you should say it in your original post! ;) -
Admin almost 11 years@MikO : Thank you, I did as you said, I changed the whole project and created classes but I get a nullPointerException, can you please check my new question : stackoverflow.com/questions/16416965/… Thnk you :)
-
B. Clay Shannon-B. Crow Raven about 10 yearsI'm having trouble grokking this; what should the getters and setters in the code above look like?
-
MikO about 10 years@B.ClayShannon, just standard getters and setters! For example:
public String getName() { return this.name; }
andpublic void setName(String name) { this.name = name; }
-
Alex Cohn almost 9 yearsTo read from file, you don't need
BufferedReader
:gson.fromJson(new FileReader(filename), Response.class)
simply works!