Parsing JSON in Express without BodyParser
Solution 1
I think the problem like to get rawBody in express.
Just like this:
app.use(function(req, res, next){
var data = "";
req.on('data', function(chunk){ data += chunk})
req.on('end', function(){
req.rawBody = data;
req.jsonBody = JSON.parse(data);
next();
})
})
And you need catch the error when parse the string to json and need to judge the Content-type
of the Req
.
Good luck.
Solution 2
another way that worked with me by collecting all chunks into an array and parsing the concatenated chunks.
app.use("/", (req, res, next)=>{
const body = [];
req.on("data", (chunk) => {
console.log(chunk);
body.push(chunk);
});
req.on("end", () => {
const parsedBody = Buffer.concat(body).toString();
const message = parsedBody.split('=')[1];
console.log(parsedBody);
console.log(message);
});
console.log(body);
});
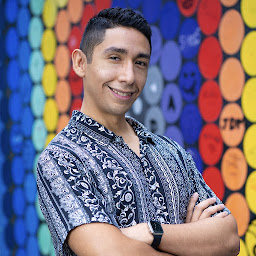
Jose
Updated on June 11, 2022Comments
-
Jose almost 2 years
I'm trying to write a simple express server that takes incoming JSON (POST), parses the JSON and assigns to the request body. The catch is I cannot use bodyparser. Below is my server with a simple middleware function being passed to app.use
Problem: whenever I send dummy POST requests to my server with superagent (npm package that lets you send JSON via terminal) my server times out. I wrote an HTTP server in a similar fashion using req.on('data')...so I'm stumped. Any advice?
const express = require('express'); const app = express(); function jsonParser(req, res, next) { res.writeHead(200, {'Content-Type:':'application/json'}); req.on('data', (data, err) => { if (err) res.status(404).send({error: "invalid json"}); req.body = JSON.parse(data); }); next(); }; app.use(jsonParser); app.post('/', (req, res) => { console.log('post request logging message...'); }); app.listen(3000, () => console.log('Server running on port 3000'));
-
Michael almost 8 yearsFor some weird reason, JSON.parse is throwing an exception because data is empty in end, but if I catch it, req.rawBody has the JSON text later.