Partially viewable bottom sheet - flutter
Solution 1
I will be implementing the same behaviour in the next few weeks and I will be referring to the backdrop implementation in Flutter Gallery, I was able to modify it previously to swipe to display and hide (with a peek area).
To be precise you can replicate the desired effect by changing this line of code in backdrop_demo.dart from Flutter Gallery :
void _handleDragUpdate(DragUpdateDetails details) {
if (_controller.isAnimating)// || _controller.status == AnimationStatus.completed)
return;
_controller.value -= details.primaryDelta / (_backdropHeight ?? details.primaryDelta);
}
I have just commented the controller status check to allow the panel to be swipe-able.
I know this isn't the complete implementation you are looking for, but I hope this helps you in any way.
Solution 2
Use the DraggableScrollableSheet
widget with the Stack
widget:
Here's the gist for the entire page in this^ GIF, or try the Codepen.
Here's the structure of the entire page:
@override
Widget build(BuildContext context) {
return Scaffold(
body: Stack(
children: <Widget>[
CustomGoogleMap(),
CustomHeader(),
DraggableScrollableSheet(
initialChildSize: 0.30,
minChildSize: 0.15,
builder: (BuildContext context, ScrollController scrollController) {
return SingleChildScrollView(
controller: scrollController,
child: CustomScrollViewContent(),
);
},
),
],
),
);
}
In the Stack
:
- The Google map is the lower most layer.
- The Header (search field + horizontally scrolling chips) is above the map.
- The DraggableBottomSheet is above the Header.
Some useful parameters as defined in draggable_scrollable_sheet.dart
:
/// The initial fractional value of the parent container's height to use when
/// displaying the widget.
///
/// The default value is `0.5`.
final double initialChildSize;
/// The minimum fractional value of the parent container's height to use when
/// displaying the widget.
///
/// The default value is `0.25`.
final double minChildSize;
/// The maximum fractional value of the parent container's height to use when
/// displaying the widget.
///
/// The default value is `1.0`.
final double maxChildSize;
Edit: Thank you @Alejandro for pointing out the typo in the widget name :)
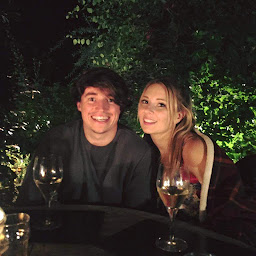
Tom O'Sullivan
Flutter Flutter Flutter. Dart. I am interested in learning Flutter (currently Beta), and will stick with it whiles it's still in production, and Dart 2.
Updated on December 07, 2022Comments
-
Tom O'Sullivan over 1 year
Is it possible in flutter to have the bottom sheet partially viewable at an initial state and then be able to either expand/dismiss?
I've included a screenshot of an example that Google Maps implements.
-
Tom O'Sullivan over 5 yearsDidn't realise you had answered this, but I saw the backdrop_demo.dart in the Flutter Gallery and had been using the
_handleDragUpdate(DragUpdateDetails details)
for my implementation by coincidence! What are the olds. Thanks for posting this and the backdrop_demo.dart. -
masewo about 4 yearsWhen using this modification in conjunction with a GoogleMap underneath then on iOS devices the _handleDragEnd does not get fired once you start swiping down the bottom sheet. Anybody does have a solution for this behaviour?
-
Alejandro over 2 yearsThere's a typo on the first sentence: it's supposed to say
DraggableScrollableSheet
insteadDraggableBottomSheet
. Just in case anyone searches for the wrong one like me ;P. By the way, I didn't know of this widget. Cool insight!