Pass an array as parameter to a controller from an ajax call in JavaScript
If you are passing an integer array properly from $.ajax
(i.e. your docsArray
should be having value like [15,18,25,30,42,49]
) then you should try :
[Authorize]
public ActionResult DeleteDocuments(int[] docsArray)
{
//int id;
//string[] arrayDocs = JsonConvert.DeserializeObject<string[]>(docsToDelete);
try {
foreach (int docId in docsArray)
{
//int.TryParse(docId, out id);
dal.DeleteDocument(docId); // dal = DataAccessLayer is the class which interacts with the database by executing queries (select, delete, update...)
}
return "Success ";
}
catch {
return "Error";
}
}
Update :
Your javascript code should be :
var sendDocsToDelete = function (docsArray) {
$.ajax({
type: 'POST',
url: 'Main/DeleteDocuments',
data: JSON.stringify(docsArray),
contentType: 'application/json; charset=utf-8',
datatype: 'json',
success: function (result) {
alert('Success ');
},
error: function (result) {
alert('Fail ');
}
});
}
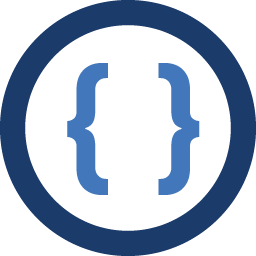
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm working on an ASP.NET MVC 4 website and I've got some troubles with a functionality. I explain, I've to select entities displayed in a table with their linked checkbox :
Screenshot of my table where each row has a checkbox with the same Id as the entity
Console showing updates in the array
Inside my script I have been abled to store each checked Id's checkbox in an array and remove those if the checkbox is unchecked. But I can't pass the array to my controller's function to delete each selected entity in the database. I used
$.ajax()
from jquery to send through a POST request the array (as JSON) but I always get 500 error :- JSON primitive invalid
- Null reference
Here's my function in my script (I don't know if my array's format is valid) :
var sendDocsToDelete = function (docsArray) { $.ajax({ type: 'POST', url: 'Main/DeleteDocuments', data: JSON.stringify(docsArray), contentType: 'application/json; charset=utf-8', datatype: 'json', success: function (result) { alert('Success ' + result.d); }, error: function (result) { alert('Fail ' + result.d); } }); }
Then, the POST call the following function in my controller :
[Authorize] [WebMethod] public void DeleteDocuments(string docsToDelete) { int id; string[] arrayDocs = JsonConvert.DeserializeObject<string[]>(docsToDelete); foreach (string docId in arrayDocs) { int.TryParse(docId, out id); dal.DeleteDocument(id); // dal = DataAccessLayer is the class which interacts with the database by executing queries (select, delete, update...) } }
Update 2
[Authorize] public ActionResult DeleteDocuments(int[] docsToDelete) { try{ foreach (string docId in arrayDocs) { int.TryParse(docId, out id); dal.DeleteDocument(id); // dal = DataAccessLayer is the class which interacts with the database by executing queries (select, delete, update...) } return Json("Success"); } catch { return Json("Error"); } } var sendDocsToDelete = function (docsArray) { $.ajax({ type: 'POST', url: 'Main/DeleteDocuments', data: docsArray, contentType: 'application/json; charset=utf-8', datatype: 'json', success: function (result) { alert('Success ' + result.d); }, error: function (result) { alert('Fail ' + result.d); } }); }
Any ideas about this issue ? I hoped I was clear enough. Do not hesitate if you need more details.
-
Admin almost 8 yearsIt have to parse it because my Ids are "checkbox123"
-
Sachin almost 8 years"checkbox123" can not be parse in
int
, you have to pass integer ids like 123 only.. you can see this : stackoverflow.com/questions/2099164/… -
Admin almost 8 yearsEach element in my array are Id defined in my view such as "checkbox123" and in the console I print each value.
-
Rajshekar Reddy almost 8 yearsI doubt that AJAX will even hit this method in the first place. Its not a ActionResult
-
Sachin almost 8 yearsThanks @Reddy, added ActionResult
-
Admin almost 8 yearsAm I maybe missing something but VS says that the ActionResult can't be an integer... Cast not working either.
-
Rajshekar Reddy almost 8 years@Loïc my bad it should be
return Json(id);
-
Admin almost 8 yearsOk it makes sense, i'll try
-
Admin almost 8 years@Sachin @Reddy So, I change Ids to be integer (in my view and when I push values in array). But I get again the exception :
System.ArgumentException - Primitive JSON invalid : undefined