pass data between Java and C
Solution 1
Provide the following declaration to your java programmer:
public native double[] doData(double [] value1, double [] value2, int count);
In your c code you will be able to fill the structure with passed parameters. JNI header will look like this:
JNIEXPORT jdoubleArray JNICALL Java_package_className_doData(JNIEnv * , jobject, jdoubleArray , jdoubleArray, jint);
Solution 2
If you want to use the same kind of "struct" on the java side I would create a class that corresponds to that struct (so that the java and the c developer can talk about the same thing):
class JavaData {
double value1[50];
double value2[50];
int count;
};
Then you need to copy the values from / to the java world when going into JNI. This is an example how you do it:
// get the class
jclass javaDataClass = env->FindClass("JavaData");
// get the field id
jfieldID countField = env->GetFieldID(javaDataClass, "count", "I");
// get the data from the field
cData.count = env->GetIntField(jData, countField);
... (the rest of the parameters)
Solution 3
While there is nothing wrong with the CSV format to receive data from different programs, I lean toward using XML to do it. Part of it is subjective preference for the data format. With XML you can define a robust construct that you can validate before you read into your program. And IMHO, a proper XML design is much more readable for debugging purposes.
Solution 4
You can probably get this to work with JNI, but personally, I'd rather have hot needles stuck in my eyes than work with JNI. I personally find Java Native Access (JNA) to be much easier to use.
Solution 5
Using a standard data container such as CSV is totally appropriate. There's tons of free Java CSV encoding libraries, and the same goes for C.
Your Java programmer can still use native Java data structures, all he/she needs to do is encode it into CSV (or other formats such as XML) and send it to you.
On your end all you have to do is read this data container using some CSV library.
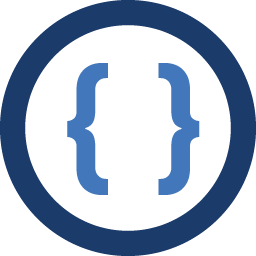
Admin
Updated on August 01, 2022Comments
-
Admin almost 2 years
I have a C structure.
struct data{ double value1[50]; double value2[50]; int count; };
I want to map data from java to this C structure.How can I do it using JNI? The java code will not be programmed by me. The java programmer just wants to know in which form should he send me the data? Should he expect any more details
I am currently testing my code by filling the structure instance with a CSV file containing 2 columns.
I also want to return 3 double values from my C code to the java application.
-
Darien over 13 yearsOnce you start having any sort of hierarchical data, I think JSON is worth considering. Of course, it may be faster to carry the data between Java and C with JNA/JNI in the form of structs...
-
Jay over 13 yearsWhy did this get voted down? I fail to see what is wrong with the post.