pass ResultSet from servlet to JSP
Solution 1
I don't understand how rows can be null
given your if
statement there.
Anyway, shouldn't it be request.getAttribute("propertyList")
in the DisplayProperties.jsp
?
Solution 2
You should also not be using a ResultSet in a JSP. That's a database cursor, a scarce resource. You may get this to "work" on a simple page, but I'd bet that you don't have clear responsibility for closing the ResultSet, Statement, or Connection in your code. You'll soon run out and wonder why your code is crashing with exceptions.
None of the java.sql interface implementations should escape out of a well-defined persistence layer. Acquire the connection, get the ResultSet, map it into an object or data structure, and close all your resources in reverse order of acquisition, then return the object or data structure to your JSP, written only with JSTL and no scriplets, for display. That's the right thing to do.
If you MUST use SQL in a JSP, use the JSTL <sql> tags to do it.
Solution 3
You've the answer, so I am only going to do an enhancement suggestion: do not use scriptlets in JSP. Use taglibs and EL where appropriate. An example to generate a list would be:
<ul>
<c:forEach items="${propertyList}" var="item">
<li>${item}</li>
</c:forEach>
</ul>
You can do the same for HTML table
s and dropdown option
s. Hope this helps.
Solution 4
Use
request.getSession().setAttribute("propertyList", Rows);
instead of
request.setAttribute("propertyList", Rows);
in your servlet code. It will work perfectly.
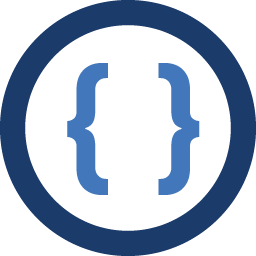
Admin
Updated on March 19, 2020Comments
-
Admin about 4 years
I am doing the following in my SampleServlet.java
//Fill resultset from db .... try { ArrayList Rows = new ArrayList(); while (resultSet.next()){ ArrayList row = new ArrayList(); for (int i = 1; i <= 7 ; i++){ row.add(resultSet.getString(i)); } Rows.add(row); } request.setAttribute("propertyList", Rows); RequestDispatcher requestDispatcher = getServletContext().getRequestDispatcher("/DisplayProperties.jsp"); requestDispatcher.forward(request,response);
and then in my jsp DisplayPropeties.jsp I have
<% ArrayList rows = new ArrayList(); if (request.getSession().getAttribute("propertyList") != null) { rows = (ArrayList ) request.getSession().getAttribute("propertyList"); } %>
but
rows
is always null.What am I doing wrong?