Pass variables between two PHP pages without using a form or the URL of page
Solution 1
Sessions would be good choice for you. Take a look at these two examples from PHP Manual:
Code of page1.php
<?php
// page1.php
session_start();
echo 'Welcome to page #1';
$_SESSION['favcolor'] = 'green';
$_SESSION['animal'] = 'cat';
$_SESSION['time'] = time();
// Works if session cookie was accepted
echo '<br /><a href="page2.php">page 2</a>';
// Or pass along the session id, if needed
echo '<br /><a href="page2.php?' . SID . '">page 2</a>';
?>
Code of page2.php
<?php
// page2.php
session_start();
echo 'Welcome to page #2<br />';
echo $_SESSION['favcolor']; // green
echo $_SESSION['animal']; // cat
echo date('Y m d H:i:s', $_SESSION['time']);
// You may want to use SID here, like we did in page1.php
echo '<br /><a href="page1.php">page 1</a>';
?>
To clear up things - SID is PHP's predefined constant which contains session name and its id. Example SID value:
PHPSESSID=d78d0851898450eb6aa1e6b1d2a484f1
Solution 2
Here are brief list:
JQuery with JSON stuff. (http://www.w3schools.com/xml/xml_http.asp)
$_SESSION - probably best way
Custom cookie - will not *always* work.
HTTP headers - some proxy can block it.
database such MySQL, Postgres or something else such Redis or Memcached (e.g. similar to home-made session, "locked" by IP address)
APC - similar to database, will not *always* work.
HTTP_REFERRER
URL hash parameter , e.g. http://domain.com/page.php#param - you will need some JavaScript to collect the hash. - gmail heavy use this.
Solution 3
<?php
session_start();
$message1 = "A message";
$message2 = "Another message";
$_SESSION['firstMessage'] = $message1;
$_SESSION['secondMessage'] = $message2;
?>
Stores the sessions on page 1 then on page 2 do
<?php
session_start();
echo $_SESSION['firstMessage'];
echo $_SESSION['secondMessage'];
?>
Solution 4
Have you tried adding both to $_SESSION
?
Then at the top of your page2.php just add:
<?php
session_start();
Solution 5
Use Sessions.
Page1:
session_start();
$_SESSION['message'] = "Some message"
Page2:
session_start();
var_dump($_SESSION['message']);
Related videos on Youtube
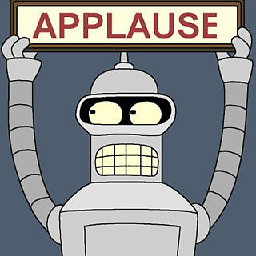
Lefteris008
Passion about science fiction, fantasy and space battles.
Updated on November 25, 2021Comments
-
Lefteris008 over 1 year
I want to pass a couple of variables from one PHP page to another. I am not using a form. The variables are some messages that the target page will display if something goes wrong. How can I pass these variables to the other PHP page while keeping them invisible?
e.g. let's say that I have these two variables:
//Original page $message1 = "A message"; $message2 = "Another message";
and I want to pass them from page1.php to page2.php. I don't want to pass them through the URL.
//I don't want 'page2.php?message='.$message1.'&message2='.$message2
Is there a way (maybe through $_POST?) to send the variables? If anyone is wondering why I want them to be invisible, I just don't want a big URL address with parameters like "&message=Problem while uploading your file. This is not a valid .zip file" and I don't have much time to change the redirections of my page to avoid this problem.
-
user2406160 about 10 yearsSessions my friend, sessions.
-