Passing a PHP Variable in external JS file
Solution 1
if you want your external javascript to be dynamic you can make it a php file and give the correct header, example:
<script type="text/javascript" src="/js/track.php"></script>
track.php
<?php
// javascript generator
Header("content-type: application/x-javascript");
?>
document.write('<IFRAME SRC="<?php echo $company['website'] ?>" WIDTH="300" HEIGHT="400"></IFRAME>');
Solution 2
PHP file (don't forget echo and quoting):
<script type="text/javascript">
var pass_this_variable = '<?php echo $company['website']; ?>';
</script>
<script type="text/javascript" src="/js/track.js"></script>
JS file (use pass_this_variable instead):
document.write('<IFRAME SRC="'+pass_this_variable+'" WIDTH="300" HEIGHT="400"></IFRAME>');
Solution 3
You should fix this line:
var pass_this_variable = <?php echo $company['website']; ?>;
Adding echo
and it should work
Solution 4
Call a PHP file inside the JavaScript source. You can find the tutorial here:
http://www.javascriptkit.com/javatutors/externalphp.shtml.
So your code will be like this:
<script type="text/javascript" src="track.php?company=<?php echo $company['website']; ?>"></script>
In the PHP file you can fetch the value through $_GET
variable and use it in the iframe. Make sure to sanitize the input.
Solution 5
JavaScript provides you the functionality of ajax for the purpose of reading the PHP or text files. Why don't you create the HTML iframe
inside a PHP file with your variables parsed and then take back the response and "throw" it inside a div.
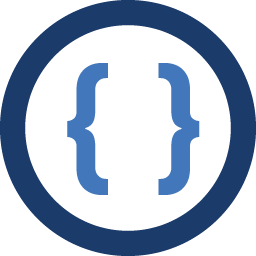
Admin
Updated on June 17, 2022Comments
-
Admin almost 2 years
I've read lots of thread on here but I am still unable to get a variable passed from PHP to an external JS file and wondered if someone could assist?
In my PHP file I have the following;
<script type="text/javascript"> var pass_this_variable = <?php $company['website']; ?>; </script> <script type="text/javascript" src="/js/track.js"></script>
In the JS file I have the following;
document.write('<IFRAME SRC="$company['website']" WIDTH="300" HEIGHT="400"></IFRAME>');
What I am trying to achieve is an IFRAME be opened and populated with what is contained within $company['website']. I know I can just use IFRAME directly in the PHP file, but this isn't what I have been tasked with for my homework. When I do use IFRAME directly in the PHP file it works fine, and if I specify a static URL in the JS file such as http://www.google.com this also works fine.
Can anyone assist? Thanks
EDIT:
Thanks for the answers so far, however I'm still unable to get it working :(
The frame that I have in track.php (or track.js) won't load the url thats specified in
$company['website']
, yet if I change it to http://www.google.com its working fine. For some reason the$company['website']
value isn't being passed :( -
Denis de Bernardy almost 13 yearsWhat if the company's name is "Joe's bar"? :-)
-
AndersTornkvist almost 13 years@Denis I believe a proper URL should be encoded with %27 instead of using single-quotes (reserved). Therefore, it won't matter in my example. However, it is always a good habit to think of line breaks, quotes etc. in strings.
-
ЯegDwight over 11 years"JavaScript provides you the functionality of ajax"? AJAX is JavaScript. That's what the J stands for.