Passing an interface instead of an object instance
Solution 1
You can have many possible implementations for that one interface. Its better to depend on an abstraction (in this case an interface) than an actual concrete class - this allows much better flexibility and testability.
This also puts the focus on what is really required by the WriteToServer()
method - the only thing its contract requires is for the caller to pass in any instance of a concrete class that provides the methods / properties declared by the IDataReader
interface.
Solution 2
Passing an interface means that you can pass any object that implements that interface not just one particular object.
This makes the code more flexible as it doesn't have to know about all the possible objects that may implement the interface now or in the future.
It also makes it more robust as it only has to deal with the properties and methods on the interface which are well known and defined.
Solution 3
The formal parameter type is an interface type - that means that you can pass in any object that implements this interface (or rather, an instance of an object that implements the interface).
You are not passing in an interface, you are passing in an object that conforms to the contract defined by the interface.
So, if your data source is SQL Server, you would pass a SqlDataReader
, if Oracle, an OracleDataReader
.
You could also implement your own data reader and pass that to the function and even write a mock data reader to test the method thoroughly.
This is a well known design principle - Program to an Interface, not an implementation.
And from MSDN - When to Use Interfaces:
Interfaces are a powerful programming tool because they let you separate the definition of objects from their implementation.
Solution 4
When a method lists one of its arguments as an interface, it isn't requesting you to pass in an instance of that interface (which is impossible anyway, you can create instances of interfaces), it's asking you to pass in any object that implements that interface.
Example:
interface IMyObject {
public void SomeMethod();
}
public class MyObject : IMyObject {
public void SomeMethod() {
// implementing code here
}
}
You can now pass any instance of MyObject as an argument that is of type IMyObject :)
public class YourObject {
public void DoSomething(IMyObject o) {
// some code here
}
}
YourObject yo = new YourObject();
MyObject mo = new MyObject();
yo.DoSomething(mo); // works
I hope that makes sense!
Solution 5
Actually, it expects you to pass an instance of a type that implements the interface, rather than the interface itself.
The interface type is used when the only thing the method cares about are the methods declared by the interface. As long as the object implements that interface, methods defined in it can be invoked on the object.
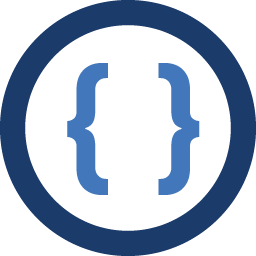
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
Take for instance the SqlBulkCopy.WriteToServer() method. One of the overloads takes an IDataReader as the parameter. My question is, what's the benefit/advantage to passing an interface to a method instead of the object instance itself?
-
Admin over 12 yearsso behind the covers of the WriteToServer() method it is calling a method (or more than one method) that the IDataReader interface defines from the object instance that is passed to that method?
-
Admin over 12 yearsthat makes absolute sense. I see that it adds quite a bit of flexibility and doesn't require a specific class as a dependency, especially with the many flavors of classes that utilize the methods of the IDataReader interface.
-
Admin over 12 yearsquestion for you. Say you wanted to use an abstract class instead of an interface (just for argument's sake). Could you have an abstract class as a parameter to a method and pass all child classes that inherit that abstract class? Why wouldn't you do that? By the way, great article on Program to an Interface, not an implementation. Definitely going to poor a cup of coffee and enjoy the rest of the read.
-
Oded over 12 years@Surfer - Yes, certainly you could use an abstract class in such a situation. Same set of arguments apply (I would say "Program to an abstraction, not an implementation"). Interfaces as a communication medium are more explicit, that's all.
-
Admin over 12 yearswhat makes them more explicit? It seems like a method from an abstract class with no implementation would be the same footprint as that from an interface.
-
Oded over 12 years@Surfer - The word... and the fact that there is no implementation at all to an interface (whereas an abstract class could have some and normally does). It is a pure contract and the users of the interface wouldn't expect or need to look at the code to find if it implements anything.