Passing data from view to controller asp.net core razor pages
Append your .cshtml file with code that outputs the values you're filling via POST.
MyPage.cshtml
@page
@model IndexModel
@using (Html.BeginForm())
{
<label for="Age">How old are you?</label>
<input type="text" asp-for="Age">
<br />
<label for="Money">How much money do you have in your pocket?</label>
<input type="text" asp-for="Money">
<br />
<input type="submit" id="Submit">
}
Money: @Model.Money
Age: @Model.Age
Now add [BindProperty]
to each property in your model, which you want to update from your OnPost()
:
[BindProperty]
public int Age { get; set; }
[BindProperty]
public decimal Money { get; set; }
Furthermore, as Bart Calixto pointed out, those properties have to be public in order to be accessible from your Page
.
The OnPost()
method is really simple, since ASP.NET Core is doing all the work in the background (thanks to the binding via [BindProperty]
).
public IActionResult OnPost()
{
return Page();
}
So now, you can click on Submit
and voila, the page should look like this:
Btw.: Properties are written with a capital letter in the beginning.
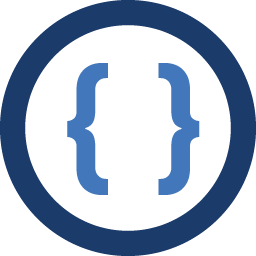
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am trying to create a simple asp.net core razor web site.
I have a cshtml page:
@page @using RazorPages @model IndexModel @using (Html.BeginForm()) { <label for="age">How old are you?</label> <input type="text" asp-for="age"> <br/> <label for="money">How much money do you have in your pocket?</label> <input type="text" asp-for="money"> <br/> <input type="submit" id="Submit"> }
and a cs file:
using Microsoft.AspNetCore.Mvc; using Microsoft.AspNetCore.Mvc.RazorPages; using System; using System.Threading.Tasks; namespace RazorPages { public class IndexModel : PageModel { protected string money { get; set; } protected string age { get; set; } public IActionResult OnPost() { if (!ModelState.IsValid) { return Page(); } return RedirectToPage("Index"); } } }
I want to be able to pass age and money to the cs file and then pass it back to the cshtml file in order to display it on the page after the submit button sends a get request. How can I implement this?
Updated: The following code does not work. index.cshtml.cs:
using Microsoft.AspNetCore.Mvc; using Microsoft.AspNetCore.Mvc.RazorPages; using System; using System.Threading.Tasks; namespace RazorPages { public class IndexModel : PageModel { [BindProperty] public decimal Money { get; set; } [BindProperty] public int Age { get; set; } public IActionResult OnPost() { /* if (!ModelState.IsValid) { return Page(); }*/ this.Money = Money; this.Age = Age; System.IO.File.WriteAllText(@"C:\Users\Administrator\Desktop\murach\exercises\WriteText.txt", this.Money.ToString()); return RedirectToPage("Index", new { age = this.Age, money = this.Money}); } } }
and index.cshtml:
@page @using RazorPages @model IndexModel @using (Html.BeginForm()) { <label for="Age">How old are you?</label> <input type="text" asp-for="Age"> <br/> <label for="Money">How much money do you have in your pocket?</label> <input type="text" asp-for="Money"> <br/> <input type="submit" id="Submit"> } Money: @Model.Money Age: @Model.Age
Money and age show up as 0s on the page and file regardless of what you type in.
-
jAC over 6 yearsAs a small info:
return RedirectToPage("SomeOtherPage", new { age = this.Age, money = this.Money});
has been there in case you wanted to redirect this info to another page. -
Admin over 6 yearsI am using an empty web site template. Your example is using a razor page template
-
jAC over 6 yearsYour example is also using Razor Pages. You tagged this post with razor-pages, your title contains razor pages and your code is a razor page (
@page
). What do you mean by empty web site template? -
Admin over 6 yearsMy code was missing the renderbody() function and the layout.cshtml, viewimports etc...