Passing Javascript Variable to Python Flask
How I did this was using an ajax request from the javascript which would look something like this. I think the easiest way would be using JQuery as well since it might be a bit more verbose with pure javascript.
// some movie data
var movies = {
'title': movie_title,
'release_date': movie_release_date
}
$.ajax({
url: Flask.url_for('my_function'),
type: 'POST',
data: JSON.stringify(movies), // converts js value to JSON string
})
.done(function(result){ // on success get the return object from server
console.log(result) // do whatever with it. In this case see it in console
})
Flask.url requires JSGlue which basically let's you use Flask's url_for but with javascript. Look it up, easy install and usage. Otherwise I think you could just replace it with the url e.g '/function_url'
Then on the server side you might have something like this:
from flask import request, jsonify, render_template
import sys
@app.route("/function_route", methods=["GET", "POST"])
def my_function():
if request.method == "POST":
data = {} // empty dict to store data
data['title'] = request.json['title']
data['release_date'] = request.json['movie_release_date']
// do whatever you want with the data here e.g look up in database or something
// if you want to print to console
print(data, file=sys.stderr)
// then return something back to frontend on success
// this returns back received data and you should see it in browser console
// because of the console.log() in the script.
return jsonify(data)
else:
return render_template('the_page_i_was_on.html')
I think the main points are to look up ajax requests in jquery, flask's request.json() and jsonify() functions.
Edit: Corrected syntax
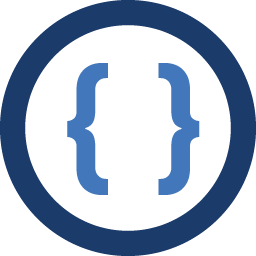
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
I have read several postings on different examples for passing a javascript variable to flask through post/get forms. I still don't understand how to do this. From my understanding, the form creates a post/get that can then be called and received by the python flask script. Can someone write up a very simple example on what this should look like?
Starting from creating a variable with any value in javascript and then making the post/get. Lastly what should the receiving end on python should look like and finally print the variable from python.