Passing parameters to a JQuery function
406,168
Solution 1
Using POST
function DoAction( id, name )
{
$.ajax({
type: "POST",
url: "someurl.php",
data: "id=" + id + "&name=" + name,
success: function(msg){
alert( "Data Saved: " + msg );
}
});
}
Using GET
function DoAction( id, name )
{
$.ajax({
type: "GET",
url: "someurl.php",
data: "id=" + id + "&name=" + name,
success: function(msg){
alert( "Data Saved: " + msg );
}
});
}
EDIT:
A, perhaps, better way to do this that would work (using GET) if javascript were not enabled would be to generate the URL for the href, then use a click handler to call that URL via ajax instead.
<a href="/someurl.php?id=1&name=Jose" class="ajax-link"> Click </a>
<a href="/someurl.php?id=2&name=Juan" class="ajax-link"> Click </a>
<a href="/someurl.php?id=3&name=Pedro" class="ajax-link"> Click </a>
...
<a href="/someurl.php?id=n&name=xxx" class="ajax-link"> Click </a>
<script type="text/javascript">
$(function() {
$('.ajax-link').click( function() {
$.get( $(this).attr('href'), function(msg) {
alert( "Data Saved: " + msg );
});
return false; // don't follow the link!
});
});
</script>
Solution 2
If you want to do an ajax call or a simple javascript function, don't forget to close your function with the return false
like this:
function DoAction(id, name)
{
// your code
return false;
}
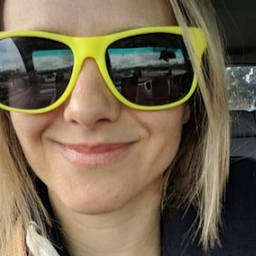
Comments
-
NicoWheat almost 2 years
I'm creating HTML with a loop that has a column for Action. That column is a Hyperlink that when the user clicks calls a JavaScript function and passes the parameters...
example:
<a href="#" OnClick="DoAction(1,'Jose');" > Click </a> <a href="#" OnClick="DoAction(2,'Juan');" > Click </a> <a href="#" OnClick="DoAction(3,'Pedro');" > Click </a> ... <a href="#" OnClick="DoAction(n,'xxx');" > Click </a>
I want that function to call an Ajax jQuery function with the correct parameters.
Any help?
-
Pim Jager almost 15 yearsYou don't have to change anything for GET, except changing TYPE to GET. Querystring will automaticly be build.
-
tvanfosson almost 15 years@Pim -- updated, thanks. I was still pretty new to jQuery when I wrote this.
-
frictionlesspulley almost 14 yearsIf you want to pass more than one parameter to the URL,use data as data:{id:'123' , name:"MyName"} where the 123 is the value of the parameter id myName is the value for the parameter name the value here can be string or variable having the value to be passed. just a simple trick to avoid string concatenation.
-
Marcus almost 14 yearsThis accepted answer looks excellent to me, but I can't help but wonder, how would this look with the onClick bindings done in jQuery instead of right in the <a> tags? I am led to believe jQuery is meant to handle the event bindings too.
-
tvanfosson almost 14 years@Marcus - the way I would actually do it is generate the URL as the href attribute. Then I'd add a click handler that gets the url from the anchor's href element and posts/gets that via ajax, returning false from the click handler. I can add that as an alternative.
-
Patricio Carvajal H. about 7 years@tvanfosson I tried the click link get href alternative you posted (I did a copy/paste), but for some reason, this line --> $.get( $(this).attr('href') do nothing (no alert), any idea?
-
tvanfosson about 7 years@PatricioCarvajalH. use the browser debugger to see if your request is failing for some reason - that would be my guess, that it's not getting back a valid response.